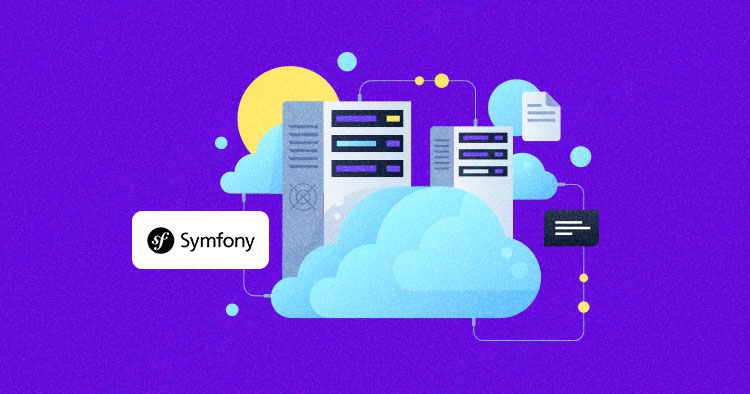
FTP is still the most used medium for transferring files between servers. A more secure version of the FTP protocol, SFTP, was introduced to ensure the security of the file transfer processes. PHP offered specific functions such as ssh2_sftp for working with PHP SFTP.
Security experts have recently detected serious problems in these functions and recommend that the developers should avoid these functions for secure file transfer. Fortunately, a more secure and reliable library named PHPSeclib, is now available for working with PHP SFTP servers. Using phpseclib SFTP results in a more secure and reliable connection that could be easily used for file transfer and related operations on any PHP web hosting.
I will demonstrate how you could set up phpseclib SFTP in Symfony and then demonstrate how you could create a secure FTP function and create, update, delete and upload files to live PHP SFTP servers.
For the purpose of this article, I assume that you have already installed Symfony 4. If not, check out how to install Symfony 4 in easy steps.
Setting up PHPseclib SFTP
Install phpseclib in Symfony
phpseclib is a secure communication library that is very compatible with the latest PHP versions. It is available as an independent package that can be integrated within Symfony through a simple installation. You can install phpseclib using Composer.
Simply go to your project folder and run the following command:
$ composer require phpseclib/phpseclib
This command will install the latest version of phpseclib in the vendor folder of Symfony project.
Since Cloudways offers a pre-integrated composer, phpseclib could be easily set up. The recommended practice is to install this PHP SFTP library through composer.json.
Open composer.json in your prefered editor and add the following code:
{ Â Â Â "require": { Â Â Â Â Â Â Â "phpseclib/phpseclib": "^2.0" Â Â Â } }
Now run composer install to install it.
Now that phpseclib is installed, I will login to my PHP SFTP server via the Master Credentials (available in the Access Details tab).
Supercharged Managed PHP Hosting – Improve Your PHP App Speed by 300%
Login to the PHP SFTP Server
For PHP SFTP server login, I will use the `defaultController.php` file.
I will initialize the phpseclib library by adding the following line at the top of the file:
use phpseclib\Net\SFTP;
Now add the following code in the `indexAction()` method of the class `DefaultController`.
$sftp = new SFTP('46.xxxx.5.xxxxx'); $sftp_login = $sftp->login('master_xxxxxxekh', 'fxxxxxh6');
Verify the login by getting the home URL of the server. For this, I will save a variable in the test.html.twig file (available in the views folder). Following is the complete code of the method:
<?php namespace AppBundle\Controller; use Sensio\Bundle\FrameworkExtraBundle\Configuration\Route; use Symfony\Bundle\FrameworkBundle\Controller\Controller; use Symfony\Component\HttpFoundation\Request; use phpseclib\Net\SFTP; class DefaultController extends Controller { Â Â Â /** Â Â Â Â * @Route("/", name="homepage") Â Â Â Â */ Â Â Â public function indexAction(Request $request) Â Â Â { $sftp = new SFTP('46.xxxx.5.23xx'); $sftp_login = $sftp->login('master_xjxxxxqsxxxh', 'f6xxxxsh6'); Â Â Â Â Â Â Â Â if($sftp_login) { Â Â Â Â Â Â Â Â Â Â Â Â Â Â return $this->render('default/test.html.twig', array( Â Â Â Â Â Â Â Â Â Â Â Â Â Â 'path' => $sftp->exec('pwd'), Â Â Â Â Â Â Â )); Â Â Â Â Â Â Â } Â Â Â Â Â Â Â else throw new \Exception('Cannot login into your server !'); Â Â Â } }
In this twig, I can print the value of the variable by adding it in between the double curly brackets {{ }}. In the test.html.twig, add the following code:
{% extends 'base.html.twig' %} {% block body %} Â {{path}} {% endblock %}
Now running the application in the browser. The home URL of the server will be displayed.
I will now describe several file operations using phpseclib.
Stop Wasting Time on Servers
Cloudways handle server management for you so you can focus on creating great apps and keeping your clients happy.
Directory Management
Using phpseclib, you can move into directories on the server and get the files. For this purpose, this PHP library provides two methods `exec()` and `chdir()`. In the `exec()` method, you can pass the ssh command and get the results.
I will now fetch the list of all the directories on the server:
Open `indexAction()` and add the following code:
public function indexAction(Request $request) Â Â Â { $sftp = new SFTP('46.xxxx.5.23xx'); $sftp_login = $sftp->login('master_xjxxxxqsxxxh', 'f6xxxxsh6'); if($sftp_login){ $dir = $sftp->exec('cd applications/wnxnxrbpgf/public_html/phpsec; ls -la'); echo "<pre>"; print_r($dir); echo "</pre>"; }
Run the application in the browser and you will get the list of all the folders on the server:
You can also get file names and information such as size, mode and permissions using chdir() method.
Add the following code in the controller:
public function indexAction(Request $request) Â Â Â { $sftp = new SFTP('46.xxxx.5.23xx'); $sftp_login = $sftp->login('master_xjxxxxqsxxxh', 'f6xxxxsh6'); if($sftp_login){ $dir = $sftp->chdir('applications/wnxnxrbpgf/public_html/phpsec'); echo "<pre>"; print_r($sftp->nlist()); print_r($sftp->rawlist()); echo "</pre>"; }
Run the application in the browser and you will get the list of all the folders on the server:
You can also get file names and information such as size, mode and permissions using chdir() method.
Add the following code in the controller:
public function indexAction(Request $request) Â Â Â { $sftp = new SFTP('46.xxxx.5.23xx'); $sftp_login = $sftp->login('master_xjxxxxqsxxxh', 'f6xxxxsh6'); if($sftp_login){ $dir = $sftp->chdir('applications/wnxnxrbpgf/public_html/phpsec'); echo "<pre>"; print_r($sftp->nlist()); print_r($sftp->rawlist()); echo "</pre>"; }
Upon execution, all the information about the files will be displayed:
You can also get the information for individual folders and files using the following methods:
$sftp->size($dir) $sftp->stat($dir) $sftp->lstat($dir)
Where `$dir` is the path of the folder on the server.
Phpseclib offers two methods `mkdir()` and `rmdir()`Â for creating and deleting files easily.
I will now create a file in my application folder by passing the URL of the project with the directory’s name.
public function indexAction(Request $request) Â Â Â { $sftp = new SFTP('46.xxxx.5.23xx'); $sftp_login = $sftp->login('master_xjxxxxqsxxxh', 'f6xxxxsh6'); if($sftp_login){ //create the directory $dir = $sftp->mkdir('applications/wnxnxrbpgf/public_html/phpsec/testfolder'); //open the directory $dir = $sftp->chdir('applications/wnxnxrbpgf/public_html/phpsec/testfolder') //remove the directory $dir = $sftp->rmdir('applications/wnxnxrbpgf/public_html/phpsec/testfolder'); }
Upon execution, all the information about the files will be displayed:
You can also get the information for individual folders and files using the following methods:
$sftp->size($dir) $sftp->stat($dir) $sftp->lstat($dir)
Where `$dir` is the path of the folder on the server.
Phpseclib offers two methods `mkdir()` and `rmdir()`Â for creating and deleting files easily.
I will now create a file in my application folder by passing the URL of the project with the directory’s name.
public function indexAction(Request $request) Â Â Â { $sftp = new SFTP('46.xxxx.5.23xx'); $sftp_login = $sftp->login('master_xjxxxxqsxxxh', 'f6xxxxsh6'); if($sftp_login){ //create the directory $dir = $sftp->mkdir('applications/wnxnxrbpgf/public_html/phpsec/testfolder'); //open the directory $dir = $sftp->chdir('applications/wnxnxrbpgf/public_html/phpsec/testfolder') //remove the directory $dir = $sftp->rmdir('applications/wnxnxrbpgf/public_html/phpsec/testfolder'); }
Similarly, to delete a single file, use the method `$sftp->delete($filepath);` where $filepath is the complete URL of the file. To delete the folder and all files in it recursively, you could use $sftp->delete($folderpath, true);
Use PHP SFTP to Upload & Download Files
Next, I will demonstrate how you can get the contents of a file or download a file from a remote server. I will use the `get()` method for this purpose:
Using PHP SFTP Upload For Multiple Files by Finder Component
In case you require PHP SFTP upload, very often you need to upload multiple files to a folder at a remote server. For this, you will need a Symfony component called finder.
First, you need to install `finder` using Composer.
$ composer require symfony/finder
Once installed, initialize the component by adding the following line of code at the top of the `defaultController.php`
use Symfony\Component\Finder\Finder;
Now in the `indexAction()` method, instantiate the object of finder and select the folder where the files to be uploaded are located. Next, I will use two methods `getRealPath()` and `getRelativePathname()` to get the file names and the relative path. Once done, I will use a `foreach` loop to upload all the files. Following is the complete code:
<?php namespace AppBundle\Controller; use Sensio\Bundle\FrameworkExtraBundle\Configuration\Route; use Symfony\Bundle\FrameworkBundle\Controller\Controller; use Symfony\Component\HttpFoundation\Request; use Symfony\Component\Finder\Finder; use phpseclib\Net\SFTP; class DefaultController extends Controller { Â Â Â /** Â Â Â Â * @Route("/", name="homepage") Â Â Â Â */ Â Â Â public function indexAction(Request $request) Â Â Â { $finder = new Finder(); $finder->files()->in(__DIR__."/testfolder"); $sftp = new SFTP('46.xxxx.5.23xx'); $sftp_login = $sftp->login('master_xjxxxxqsxxxh', 'f6xxxxsh6'); if($sftp_login){ foreach ($finder as $file) { print_r($sftp->put('/applications/wnxnxrbpgf/public_html/phpsec'.$file->getRelativePathname(),$file->getRealPath(),SFTP::SOURCE_LOCAL_FILE)); } } }
Conclusion
In this piece, I have discussed how you can create a secure PHP SFTP connection with the Cloudways server and perform basic CRUD actions for files and folders. You can also try this library for your servers and extend it for your project’s purposes. Refer to official documentation of phpseclib for help. Setting up PHP SFTP with phpseclib is the best way of implementing a secure file transfer process.
If you have any query or suggestions, please leave a comment below!
Q. Which PHP editor comes with SFTP support ?
A: There are a number of PHP editors available in the market which not only support FTP, but also support SFTP. Among the most popular editors that support SFTP are Eclipse, Netbeans, jEdit, Bluefish and a few others.
Q. How to Connect PHP SFTP with key ?
A: You can easily connect SFTP with PHP using PHPSeclib. The library is precisely made to facilitate PHP SFTP connection with key. Just pass the key in place of password to the login method of SFTP and the connection is made successfully.
Q. PHP FTP vs SFTP: Which one is better ?
A: The major difference between FTP and SFTP is the authentication system. SFTP is a much more secure protocol as compared to FTP and provides hassle-free file transferring with security. FTP uses multiple port numbers for communication, whereas SFTP only uses one, which is what makes it more secure than FTP.