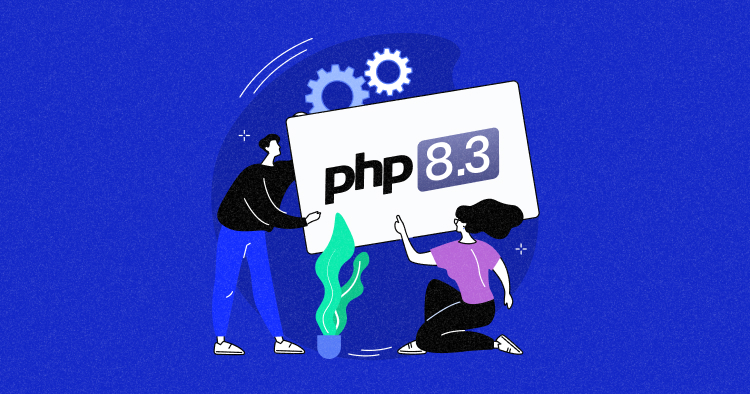
Disclaimer:Â This is a guest blog submitted by Kane
As the PHP project adheres to its annual tradition of releasing a new major or minor version towards the end of each year, PHP 8.3 is no exception.
Exciting news for developers and businesses alike! Cloudways is excited to announce support for PHP 8.3 on our Cloudways Flexible hosting plans. This latest PHP version promises enhanced performance, and improved security features to ensure your applications run smoother and faster than ever before.
Released on November 23, this latest iteration brings forth many new features and enhancements, further solidifying PHP’s standing as a versatile and evolving language.
In this article, we will delve into the noteworthy additions and changes introduced in PHP 8.3, shedding light on the key aspects developers need to be aware of.
Unleash the Power of PHP: Lightning-fast Hosting, Seamless Performance!
Experience the seamless blend of power, reliability, and simplicity that makes Cloudways the go-to choice for PHP hosting enthusiasts worldwide.
New Additions to PHP 8.3
1. Typed Class Constants
PHP 8.3 introduces a significant enhancement in the form of typed class constants. With this addition, developers can now declare types for class constants, ensuring type compatibility and reducing the likelihood of unintended deviations from the initial declaration. This enhancement extends to interface, trait, and enum constants as well.
interface ConstTest { const string VERSION = "PHP 8.3"; } // Illegal: interface ConstTest { const float VERSION = "PHP 8.3"; }
Typed class constants prove valuable in maintaining type consistency when working with child classes derived from base declarations, preventing accidental changes to the type that may lead to compatibility issues.
2. json_validate() Function
Prior to PHP 8.3, determining the syntactic validity of a JSON-encoded string involved using json_decode() and checking for errors.
The new json_validate() function simplifies this process by validating the JSON syntax without the need to construct associative arrays or objects, thereby conserving memory.
php if (json_validate($maybeJSON)) { // Do something with $maybeJSON }
This function is particularly useful for validating JSON payloads before storage or delivery in a request-response.
3. Dynamic Class Constant and Enum Number Fetch Support
In PHP 8.3, fetching class constants and enum members with variable names becomes more straightforward. The cumbersome use of the constant() function is replaced with a more intuitive syntax, enhancing code readability.
php $constantName = 'THE_CONST'; $memberName = 'FirstMember'; echo MyClass::{$constantName}; echo MyEnum::{$memberName}->value;
This improvement simplifies the process of accessing class constants and enum members dynamically.
4. gc_status() Now Returns Additional Information
The gc_status() function, responsible for providing garbage collector statistics, receives an upgrade in PHP 8.3. The function now returns additional information, including details about the running status, protection status, buffer size, and more.
php $gcStatus = gc_status(); echo "Running: " . $gcStatus['Running']; echo "Memory usage before collection: ".$gcStatus['memoryUsageBefore']; echo "Memory usage after collection: " .$gcStatus['memoryUsageAfter'];
This enhancement provides developers with a more comprehensive view of the garbage collector’s behavior.
5. New \Random\Randomizer::getBytesFromString Method
PHP 8.3 introduces the getBytesFromString method in the \Random\Randomizer class, enabling the generation of random sequences based on a specified string of characters. This method proves useful for generating secure random bytes from a predefined set of characters.
php $rng = new Random\Randomizer(); $alpha = 'ABCDEFGHJKMNPQRSTVWXYZ'; $rng->getBytesFromString($alpha, 6); // "MBXGWL" $rng->getBytesFromString($alpha, 6); // "LESPMG" $rng->getBytesFromString($alpha, 6); // "NVHWXC"
This method adds flexibility to random data generation by allowing developers to specify the source material for random byte selection.
6. New \Random\Randomizer::getFloat() and nextFloat() Methods
Expanding on the Random extension, PHP 8.3 introduces two new methods for generating random float values: getFloat() and nextFloat(). These methods enhance the precision and control over the generation of random floating-point numbers.
php $rng = new Random\Randomizer(); // Generate a float value between 0 and 5 $rng->getFloat(0, 5); // 2.3937446906217
These methods provide developers with more options for obtaining random float values within specified ranges.
7. Fallback Value Support for PHP INI Environment Variable Syntax
In the 8.3 version, PHP developers can specify fallback values for PHP INI settings when certain environment variables are absent, providing default values for greater configurability.
php // Fallback to 'Foo' if SESSION_NAME is not set session.name = ${SESSION_NAME:-Foo} // Fallback to '[email protected]' if MAIL_FROM_USER or MAIL_FROM_DOMAIN is not set sendmail_from = "${MAIL_FROM_USER:-info}@${MAIL_FROM_DOMAIN:-example.com}"
This addition simplifies configuration management by allowing graceful handling of missing environment variables.
8. PHP CLI Lint Supports Linting Multiple Files at Once
A notable improvement in PHP 8.3 is the ability to lint multiple files in a single invocation of the PHP CLI linting process. This enhancement streamlines the linting workflow, providing more efficiency when checking syntax errors across multiple files.
bash php -l file1.php file2.php file3.php
This update improves the developer experience by allowing simultaneous linting of multiple files.
9. class_alias() Supports Aliasing Built-in PHP Classes
In PHP 8.3, the class_alias() function gains the capability to alias built-in PHP classes. Developers can now use class_alias() to create aliases for core PHP classes, providing more flexibility in code organization and naming conventions.
php class_alias(\DateTime::class, 'MyDateTime'); $customDateTime = new MyDateTime();
This enhancement allows for cleaner and more expressive code when working with built-in PHP classes.
10. New stream_context_set_options Function
PHP 8.3 introduces a new function, stream_context_set_options, as an improvement over the existing stream_context_set_option. The new function supports multiple options and is expected to replace the original function in future PHP versions.
php stream_context_set_options($stream_or_context, ['http' => ['method' => 'POST']]);
This update enhances stream context manipulation by providing a more versatile and future-proof API.
Host PHP Websites with Ease [Starts at $11 USD/Mon]
- Free Staging
- Free backup
- PHP 8.2
- Unlimited Websites

Deprecations and Changes in PHP 8.3
1. get_class() and get_parent_class() Changes
In PHP 8.3, calling get_class() and get_parent_class() without parameters is deprecated. This change aims to streamline the usage of these functions and eliminate potential ambiguities arising from multiple function signatures.
php class MyException extends InvalidArgumentException { public function __construct() { get_class(); // Deprecated in PHP 8.3 get_parent_class(); // Deprecated in PHP 8.3 } }
Developers are encouraged to provide the $object parameter when using these functions to avoid deprecation notices.
2. unserialize(): E-NOTICE to E-WARNING
The behavior of the unserialize() function undergoes a change in PHP 8.3, where certain error conditions that previously emitted notices (E_NOTICE) now emit warnings (E_WARNING). This includes cases such as syntax errors and failures in custom __unserialize handlers.
php // PHP 8.3 unserialize('invalid_data'); // Now emits E_WARNING for syntax errors
This change promotes consistency in error handling and encourages developers to handle unserialized errors more proactively.
3. HTML Highlight Tag Changes
The syntax highlighting functions in PHP, namely highlight_file and highlight_string, undergo changes in PHP 8.3. The resulting HTML output is now wrapped in <pre><code></code></pre>, and line breaks are no longer converted to <br /> tags. Additionally, white spaces and tabs are no longer converted to HTML entities.
php $code = file_get_contents('example.php'); echo highlight_string($code, true);
This adjustment improves the clarity of highlighted code and aligns with modern HTML standards.
4. Granular DateTime Exceptions
PHP 8.3 introduces granular Exception and Error classes specific to date-related issues, providing more detailed information in case of errors. These extension-specific classes enhance the precision of error reporting related to DateTime operations.
php try { // DateTime-related operation } catch (\DateTimeException $e) { // Handle DateTime-specific exception } catch (\Exception $e) { // Handle other exceptions }
This refinement in exception handling aids developers in identifying and addressing date-related errors more effectively.
How to Upgrade to the Latest PHP Version Cloudways?
You can easily upgrade your current PHP version to the latest version on Cloudways by following the steps below:
- Login to the Cloudways Platform.
- Select your server.
- You’ll be redirected to the Server Management page.
- Click Settings & Packages > Packages.
- Select PHP 8.3 from the drop-down menu.
- Click Save.
- That’s how easily you can upgrade your PHP version on Cloudways.
Cloudways: Where PHP Hosting Meets Unrivaled Speed and Security!
Elevate Your PHP Projects with Cloudways: Where Speed Meets Simplicity. Take Your PHP Development to New Heights with Cloudways’ Hosting Solutions.
Why Business Owners and Agencies Need PHP 8.3
Upgrading to PHP 8.3 is a game-changer for business owners and agencies. With significant performance improvements, your websites and applications will load faster, providing a better user experience and potentially increasing conversion rates. Enhanced security features offer better protection against vulnerabilities, ensuring your data and your customers’ data remain safe.
By upgrading to PHP 8.3 on Cloudways Flexible, you can ensure your web applications stay ahead of the curve, delivering an exceptional user experience while prioritizing security with new functionalities and streamline development processes, enabling your team to deliver projects more efficiently and cost-effectively.
Conclusion
While PHP 8.3 may not introduce groundbreaking features comparable to previous versions like PHP 8.0 and 8.1, it focuses on refining the language, aligning features with evolving industry standards, and addressing various aspects of syntax and error handling.
Developers and teams familiar with these enhancements can leverage them wisely to improve code quality and maintainability.
If you’re considering transitioning to PHP 8.3, a thorough review of the documentation is recommended to ensure a smooth adaptation to the new features and changes.
In the top backend frameworks of the 2024 category, one name stands out above the rest – PHP with its dynamic Laravel framework. With PHP and Laravel, the possibilities are truly limitless.
And remember, you can upgrade to the latest version of PHP right from the Cloudways platform in just a few clicks.
Q. Is PHP 8.3 Now Out?
A: Yes, PHP 8.3 was released as scheduled on November 23, introducing numerous features and improvements since PHP 8.2.
Q. What’s New in PHP 8.3?
A: PHP 8.3 is a significant update, featuring explicit typing of class constants, deep-cloning of readonly properties, and additional randomness functionality. Alongside new features, it includes performance enhancements, bug fixes, and general cleanup.
Author’s Bio
![]() |
Kane helps clients by transforming businesses through innovative ideas on their digital journey. Currently, he works at Ace Infoway. It’s no secret that he really loves writing meaningful content, where he perspectively talks about Software development, new ideas, future technologies, and SEO. |