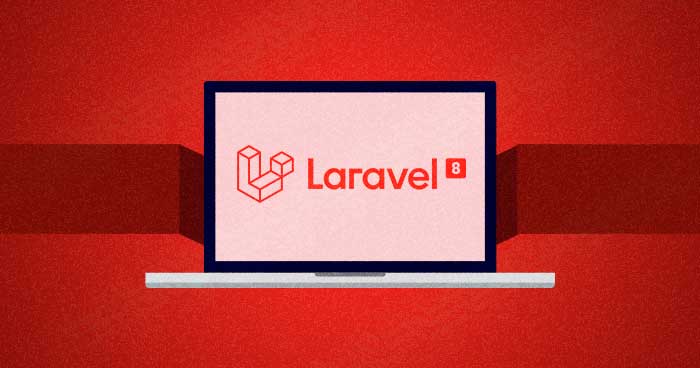
Laravel 8 was made available on September 8, 2020. It comes with exciting features and improvements, including Laravel Jetstream, a model directory, rate-limiting improvements, dynamic blade components, and others. It has an expressive, elegant syntax and provides the right tools needed for large, robust applications. Laravel 8 also offers an advanced alternative for Symfony, which is not intended for built-in user authorization.
Laravel 8 provides cool features that add to a PHP developer’s adaptability. All the combined tools and features make a difference in building everything from scratch, whether simple websites or large-scale applications.
This blog will walk you through the easy steps to install Laravel on the Cloudways Platform. But first, let’s check out Laravel 8’s improvements and new features so you can start using it to streamline your projects.
Nothing as Easy as Deploying Laravel Apps on Cloud
With Cloudways, you can have your PHP apps up and running on managed cloud servers in just a few minutes.
What’s New in Laravel 8 – Features and Improvements
Laravel 8 has a modern landing page for an innovative installation process compared to Laravel 7. In Laravel 7, the RouteServiceProvider had an attribute called namespace that was utilized to prefix the controllers within the routes files.
Laravel gives more security to core PHP through libraries, providing verified and secure code and extra security features such as an authentication framework, tokens to avoid third-party attacks, and security against cross-site scripting SQL injections.
Let’s see what we have new in Laravel 8:
Laravel Jetstream
Laravel Jetstream has improved the existing Laravel UI scaffolding available in earlier versions. It offers a simple initiating point for new projects. It also provides pre-built components including user registration and login, two-factor authentication, email verification, API support via Laravel, session, and team management.
Models Directory
The application structure in Laravel 8 includes an app/Models directory. Every command now assumes that the models are available in the app/Models. If this directory isn’t found, the framework will consider that the application models are available within the app/ folder.
Rate Limiting Improvement
Rate limiting has been improved in Laravel 8 with support for backward compatibility with existing throttle middleware and much better flexibility. You can now define the concept of Rate Limiters through a façade;
use Illuminate\Cache\RateLimiting\Limit; use Illuminate\Support\Facades\RateLimiter; RateLimiter::for('global', function (Request $request) { return Limit::perMinute(1000); });
Note that the method for() takes the HTTP request instance, giving you full control over dynamically limiting the requests.
Time Testing Helpers
The powerful Carbon PHP library now allows complete control over time modification. Laravel 8 further improves it by allowing users convenient test helpers for manipulating the time within tests.
Note that when you are using the following methods, the time will reset in between each test;
// Future $this->travel(5)->milliseconds(); $this->travel(5)->seconds(); $this->travel(5)->minutes(); $this->travel(5)->hours(); $this->travel(5)->days(); $this->travel(5)->weeks(); $this->travel(5)->years(); // Past $this->travel(-5)->hours(); // Current Time $this->travelTo(now()->subHours(6)); // Present $this->travelBack();
Model Factory Classes
In Laravel 8, Eloquent model factories are now class-based with improvement in support for relationships between factories.
The model factories exceptionally valuable for testing and seeding fake data into your database. This allows you to see your code in activity before it records any real user data.
Here’s the new syntax for the generation of records via the improved model factories.
use App\Models\User; User::factory()->count(50)->create(); // using a model state "suspended" defined within the factory class User::factory()->count(5)->suspended()->create();
Dynamic Blade Components
Laravel 8 lets you render a blade component in runtime, which wasn’t available in the previous versions.
Migration Squashing
With Laravel 8, you can squash multiple migration files in your application directory into a single SQL file. While running migrations, this file will be executed first, followed by any remaining migration files that are not part of the file. Squashing existing migrations helps decrease the migration file’s bloat and improve the performance while running tests.
Artisan serve Improvements
Previously, the command required you to manual stop and restart. In Laravel 8, the Artisan server command is improved with auto-reloading upon detection of environment variable changes in the local .env file.
Job Batching
This feature allows you to swiftly run a batch of jobs and then perform actions when the batch is completely executed. The new batch method of the bus facade can be used to dispatch a batch of jobs.
Batching is useful when coupled with completion callbacks. As such, you can use the then, catch, and finally methods to define the completion callbacks.
Each of the callbacks will receive an Illuminate\Bus\Batch instance when invoked.
Bus::batch([ new ProcessFile(1), new ProcessFile(2), new ProcessFile(3), ])->dispatch();
To learn more details about job batching, you can visit the Laravel’s queue documentation
Laravel 8: Changes and New Features in Queue System
Now that we’ve covered the essential features of Laravel 8, let’s dive into the Laravel 8’s queue system changes, updates, and new features.
Backoff
The retryAfter Property and retryAfter() function of queued mailers, jobs, notifications, and listeners has been renamed to backoff.
The php artisan queue:work command’s option –delay is also renamed to –backoff.
Job Expiration
The timeoutAt property of queued jobs, listeners, and notifications has been renamed to retryUntil. It instructs the worker to continue trying until a specific time.
You can use retryUntil as a retryUntil method or as a public property on the job.
Public function retryUntil () { Return now()->addDay(); }
Queued Closure
While dispatching a queued closure, you can now chain a catch() method;
Dispatch(function () { // Job Logic })->catch(function (Throwable $e) { // Handle Failure });
If the job fails then the catch() method will be invoked.
Database Driver Reliability
Laravel 8 lets you perform activities like releasing a job back into the queue via queue driver for the database inside a transaction. So, the job won’t be removed from the queue until the new instance — released — is inserted, reducing the risk of losing employment.
Redis Driver Efficiency
Laravel can operate while dispatching a group of jobs in bulk using the Redis queue driver by sending a single command to Redis. With earlier versions, Laravel used to send multiple rpush commands to Redis, one for each task.<“>Worker Termination
With Laravel 8 and further, workers will gracefully terminate and call any exiting callbacks registered by App:terminating().
Worker Self Termination
In previous Laravel versions, terminating PHP workers and letting the monitoring tools start new ones was a common practice to restrict memory leaks.
This practice was earlier done by adding a CRON job that runs the queue:restart artisan command. With Laravel 8, you can define instructors to exit after processing numbers or seconds of the job.
php artisan queue:work --max-jobs=1000 --max-time=3600
Naming the Workers
Now you can add a –name option to the queue:work command:
php artisan queue:work –name=notifications
This functionality has been mainly added to allow individuals to customize how the worker chooses which queue to process jobs at run time:
Worker::popUsing('notifications', function ($pop) { $queues = time()->atNight() ? ['mail', 'webhooks'] : ['push-notifications', 'sms', 'mail', 'webhooks']; foreach ($queues as $queue) { if (! is_null($job = $pop($queue))) { return $job; } } });
Horizon Balancing Rate
We now have two new configuration options to Horizon;
balanceMaxShift and balanceCooldown
'environments' => [ 'environment' => [ 'supervisor-1' => [ 'balanceMaxShift' => 5, ], ], ],
balanceMaxShift sets the maximum number of processes to be added or removed each time Horizon scales the pool of workers. Just a single worker has been added or removed in previous versions on Horizon now you can monitor that number.
balanceCooldown sets the number of seconds to wait between each scaling action. In earlier versions of Horizon, this was hard-coded to 3 seconds.
'environments' => [ 'environment' => [ 'supervisor-1' => [ 'balanceCooldown' => 1, ], ], ],
Server Prerequisites to Install Laravel 8
You will need to make sure your server meets the following requirements:
- PHP Version 7.3
- BCMath PHP Extension
- Ctype PHP Extension
- Fileinfo PHP extension
- JSON PHP Extension
- Mbstring PHP Extension
- OpenSSL PHP Extension
- PDO PHP Extension
- Tokenizer PHP Extension
- XML PHP Extension
Install Laravel 8 on Cloudways Platform
To Install Laravel on the server, select your server and application according to your project requirements. Select Laravel server size as per your website traffic, and location according to your preferred region.
After completing all these steps, you can finally navigate to the application tab and select the application.
Starting the Laravel installation process on the server requires you to open the SSH terminal to run the composer command. You can use the Putty terminal or Cloudways SSH terminal.
Improve Your Laravel App Speed by 300%
Cloudways offers you dedicated servers with SSD storage, custom performance, an optimized stack, and more for 300% faster load times.
Let’s start with Putty: go to the application public_html folder and type the following Laravel installation command:
composer create-project --prefer-dist laravel/laravel blog
Now, go to your application path: APPLICATION-URL/laravel/public, where you will see the following screen.
Conclusion
This article covers all of Laravel 8’s latest features to help you get started and also guides you on installing Laravel using the composer.
Laravel 8 has resolved many minor bugs encountered in earlier versions and added new functionalities for the Laravel community.Â
Since the announcement of Laravel 8’s release in Laracon, it has only received positive feedback from the industry. However, as the dev community digs in deep and migrates to the new features-rich Laravel 8, we’ll see how these features and the overall framework actually perform. You can also read more about Laravel 9’s new features.
Please provide your feedback on the features mentioned above and share your experiences with Laravel 8 in the comments below.
Q: How do I install Laravel?
A: Start by installing Composer:
Next, download Laravel through the following Composer command:
composer global require "laravel/installer=~1.1"
Once done use the following command to install Laravel:
composer create-project laravel/laravel 4.2 --prefer-dist
Q: How do I install the latest version of Laravel?
A: Use the following command:
composer create-project laravel/laravel="x"
Where x is the version you wish to install
Q: How do I get started in Laravel?
A: The first requirement is to set up Laravel so that you have the right dev environment for your projects. Next, opt for free and paid trainings that give you a head start in learning Laravel.
Salman Siddique
Salman is a content marketer with a CS background, so he is (very) good at creating and marketing technical content. When not working as a Digital Content Producer at Cloudways, he enjoys reading interesting stuff and learning new skills.