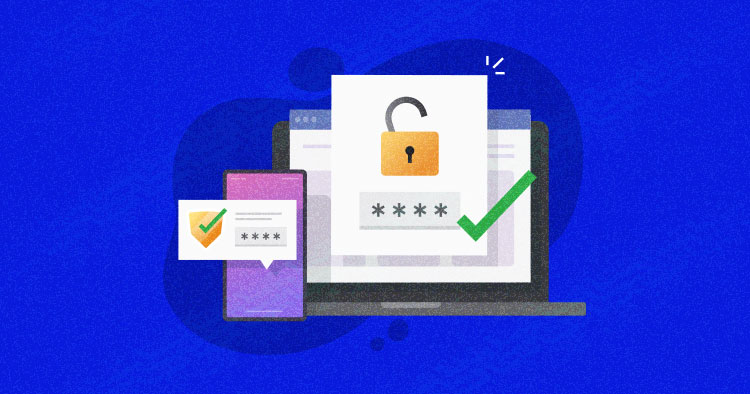
Picture a scenario where your application demands a reliable and secure login system to protect user data, restrict access to authorized users, and provide a seamless user experience. Laravel, renowned for its elegant syntax and comprehensive features, offers a range of authentication methods to fit your specific project requirements.
With Laravel’s built-in authentication functionalities and powerful packages, you can create a solid foundation for user authentication without reinventing the wheel. Elevate your security measures with the prowess of managed Laravel hosting, ensuring a seamless user experience.
This blog will explore the key concepts and implementation steps for setting up Laravel login authentication and best practices to help you establish a secure and user-friendly login system in no time.
Different Approaches to Laravel Authentication
When it comes to setting up login authentication in Laravel, there are several methods available that cater to different authentication requirements.
Laravel, being a powerful PHP framework, provides developers with flexibility and options to choose the authentication method that best suits their project’s needs.
Managed Laravel Hosting: Elevate Your Web Experience
Say goodbye to hosting complexities. Cloudways’ managed Laravel hosting takes care of the technicalities, so you can focus on crafting remarkable web experiences.
Let’s explore a couple of the most commonly used Laravel authentication methods.
Password Based Authentication
Password-based authentication is a commonly used method for user authentication, where users verify their identity by providing a username and password. Laravel, being a versatile PHP framework, provides a straightforward way to set up password-based authentication using its built-in features.
To setup password-based authentication, you need to ensure you have Laravel 9 installed on your system. Once you have a Laravel project set up, follow these steps:
- Start by configuring your database connection in the .env file located at the root of your Laravel project.
- Set the appropriate values for DB_CONNECTION, DB_HOST, DB_PORT, DB_DATABASE, DB_USERNAME, and DB_PASSWORD according to your database setup.
- Run the following command to generate the migration file:
php artisan make:migration create_users_table --create=users
- You can define the necessary fields for the users table, Once you have defined the table structure, run the migration to create the users table
php artisan migrate
- Laravel’s User model represents the users table. Make sure the User model exists in the app/Models directory or the default app directory if you’re using an older Laravel version.
- If it doesn’t exist, you can generate it using the following command
php artisan make:model Models\\User
- open the config/auth.php file and configure the authentication guards and providers. Make sure that the default guard is set to web
'defaults' => [ 'guard' => 'web', 'passwords' => 'users', ],
- Access the registration page by visiting /register on your Laravel application’s URL.
- Visit the login page at /login to log in with the registered user’s credentials. Laravel’s authentication system will handle the verification process and create a session for the authenticated user
Token Based Authentication
Token-based authentication is a popular method for securing APIs and providing stateless authentication. Instead of relying on sessions or cookies, tokens are used to authenticate and authorize API requests.
Laravel 9 offers built-in support for token-based authentication through the Laravel Sanctum package.
Here’s a step-by-step guide to implementing token-based authentication in Laravel:
- Start by installing the Laravel Sanctum package using Composer.
composer require laravel/sanctum
- Next, run the migration to create the necessary tables for Sanctum:
php artisan migrate
- To enable Sanctum, you need to update your app/Http/Kernel.php file. Add the \Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class middleware to the $middlewareGroups array:
'api' => [ \Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class, 'throttle:60,1', \Illuminate\Routing\Middleware\SubstituteBindings::class, ],
- Generate API Tokens
php artisan sanctum:tokens
- Enable Token Authentication for Users
use Laravel\Sanctum\HasApiTokens;
class User extends Authenticatable { use HasApiTokens; // ... }
- Authentication Routes and Middleware; To generate these, run the following command:
php artisan vendor:publish --provider="Laravel\Sanctum\SanctumServiceProvider"
This will publish the Sanctum configuration file, migration, and routes. You can find the routes in the routes/api.php file.
- To secure your API routes, you can apply the Sanctum middleware to them.
'auth:sanctum' => \Laravel\Sanctum\Http\Middleware\EnsureFrontendRequestsAreStateful::class,
- Now you can use the auth:sanctum middleware to protect your API routes.
Multi-Factor Authentication
Multi-factor authentication (MFA) adds an extra layer of security to user authentication by requiring users to provide multiple forms of verification.
This approach significantly enhances the security of user accounts by combining something the user knows with something they possess or something unique to them such as a fingerprint or facial recognition.
Laravel 9 provides built-in support for implementing multi-factor authentication through the Laravel Fortify package.
Here’s a step-by-step guide to setting up multi-factor authentication in Laravel:
- Start by installing the Laravel Fortify package using Composer
composer require laravel/fortify
- Next, run the migration to create the necessary tables for Fortify:
php artisan migrate
- Enable multi-factor authentication:
'features' => [ // ... 'two-factor-authentication' => true, ],
- Make sure the model implements the TwoFactorAuthenticatable contract and imports the Laravel\Fortify\TwoFactorAuthenticatable trait:
use Laravel\Fortify\TwoFactorAuthenticatable; use Illuminate\Contracts\Auth\MustVerifyEmail; use Illuminate\Foundation\Auth\User as Authenticatable; use Illuminate\Notifications\Notifiable; class User extends Authenticatable implements MustVerifyEmail { use Notifiable, TwoFactorAuthenticatable; // ... }
- Enable two-factor authentication for users:
'two-factor' => [ 'providers' => [ 'users' => [ 'driver' => 'eloquent', 'model' => App\Models\User::class, ], ], ],
- Generate Laravel pre-built views and routes for multi-factor authentication using the following command:
php artisan fortify:two-factor-auth
This command generates the necessary views in the resources/views/auth/two-factor directory and adds the required routes to your routes/web.php file.
- Customize the authentication process, by modifying the views in resources/views/auth/two-factor and the corresponding controllers.
Prerequisites for Laravel 9.X Custom Authentication
- To begin, get your server on Cloudways if you do not have one.
- Launch a Laravel 9.x app.
Step 1: Setup the Database
- Go to your Laravel application on the Cloudways server.
- Click on Launch Database.
- Click on Create Table. Name the new table users and select innoDB and ascii_general
- Add the following columns in the table:
- id (int) (Check AutoIncrement A.I.)
- name (text)
- username (text)
- email (text)
- password (text)
- remember_token (text)
- timestamp (time)
- Click Save
- Now Click New Item and then add the following user:
- Name: Cloudways user
- Username: NewUser
- Email: [email protected]
- Password: Start123
Click Save
Tailor Made Cloud Hosting For Different PHP Frameworks
From Laravel to CodeIgniter, We Have Got You Covered On All PHP Frameworks!
Step 2: Setup the Routes
your-project-root-directory/routes/web.php
Here lies all the endpoints.
Let’s make three endpoints:
- Post Call to submit the form
- Get Call to show the login form
- To LogOut.
<?php /* |-------------------------------------------------------------------------- | Application Routes |-------------------------------------------------------------------------- */ // route to show the login form Route::get('login', array( 'uses' => 'MainController@showLogin' )); // route to process the form Route::post('login', array( 'uses' => 'MainController@doLogin' )); Route::get('logout', array( 'uses' => 'MainController@doLogout' )); Route::get('/', function () { return view('welcome'); });
Step 3: Make the Controllers
Using the following command to read information from the Request object and creates and returns a Response object.
use App\Http\Controllers\UserController;
Route::get('/user/{id}', [UserController::class, 'show']);
I will extend MainController from the Base Controller and make three functions to show login, check the login and for logging out.
<?php namespaceAppHttpControllers; useRedirect; useAuth; useInput; useIlluminateSupportFacadesValidator; useIlluminateFoundationBusDispatchesJobs; useIlluminateRoutingController as BaseController; useIlluminateFoundationValidationValidatesRequests; useIlluminateFoundationAuthAccessAuthorizesRequests; useIlluminateFoundationAuthAccessAuthorizesResources; useIlluminateHtmlHtmlServiceProvider; class MainController extends BaseController { public function showLogin() { // Form View return view('login'); } public function doLogout() { Auth::logout(); // logging out user return Redirect::to('login'); // redirection to login screen } public function doLogin() { // Creating Rules for Email and Password $rules = array( 'email' => 'required|email', // make sure the email is an actual email 'password' => 'required|alphaNum|min:8' // password has to be greater than 3 characters and can only be alphanumeric and); // checking all field $validator = Validator::make(Input::all() , $rules); // if the validator fails, redirect back to the form if ($validator->fails()) { return Redirect::to('login')->withErrors($validator) // send back all errors to the login form ->withInput(Input::except('password')); // send back the input (not the password) so that we can repopulate the form } else { // create our user data for the authentication $userdata = array( 'email' => Input::get('email') , 'password' => Input::get('password') ); // attempt to do the login if (Auth::attempt($userdata)) { // validation successful // do whatever you want on success } else { // validation not successful, send back to form return Redirect::to('checklogin'); } } } }
Step 4: Setup the View
Now let’s make the view, which will contain the HTML code of the app.
$ vim resources/views/checklogin.blade.php
The .blade.php extension lets Laravel know that I am using its Blade Templating system.
Here is the HTML code for this file.
<!doctype html> <html> <head> <title>My Login Page</title> </head> <body> < {{ Form::open(array('url' => 'login')) }} <h1>Login</h1> <!-- if there are login errors, show them here --> <p> {{ $errors->first('email') }} {{ $errors->first('password') }} </p> <p> {{ Form::label('email', 'Email Address') }} {{ Form::text('email', Input::old('email'), array('placeholder' => '[email protected]')) }} </p> <p> {{ Form::label('password', 'Password') }} {{ Form::password('password') }} </p> <p>{{ Form::submit('Submit!') }}</p> {{ Form::close() }}
Now let’s check how the app looks!
This is a simple example of how you could implement login authentication in a Laravel app.
Another Laravel Login and Registration Setup
Here is a GIF that explains the entire process:
Database Migration
In a Laravel powered app, database configuration is handled by two files: env and config/database.php. In my case, I created a database with the name loginuser. The Cloudways Database Manager makes the entire process very easy.
Next, run the following command in the terminal to create tables in the database:
Login to SSH terminal by using Master Credentials and go to the application folder by using the command
$ ls applications $ cd applications applications$ ls pjbeasusxr trxbnbphae applications$ cd trxbnbphae/ trxbnbphae$ cd public_html/
php artisan migrate
Now, when you check the database, you will see that the tables have been created successfully.
Setup Laravel Authentication
php artisan make:auth
This can also be used as for routes for all authentication end-points.
Register
Login
Use Username for Authentication
Laravel uses the the default email address as authentication field. However, users prefer to use their username instead of emails. To change the default behavior, here is how to define a username in the controller.
Login Controller
public function username() { return 'username';}
Empower Your Laravel Projects With Cloudways’ Laravel Managed Hosting!
Unleash the Power of Laravel with Managed Hosting – Where Innovation Meets Effortless Excellence!
Conclusion
Setting up Laravel login authentication doesn’t have to be a daunting task. With the right approach and utilizing the powerful features you can implement a strong and secure authentication system for your web application.
Remember to consider your project’s specific needs and security requirements when choosing the authentication method. And if you have any questions, leave them in the comments and I’ll get back to ASAP.
What is authentication in Laravel?
Authentication is the process of identifying the client credentials. In web applications, authentication is overseen by sessions that take the input parameters such as mail or username and password, for client recognizable proof.
How do I enable authentication in Laravel?
You need to Install the laravel/ui Composer bundle and run php artisan ui vue –auth in a new Laravel application. After migrating your database, open http://your-app.test/register or any other URL that’s assigned to your application on your browser.
Q) What are the different authentication methods available in Laravel?
A) Laravel provides various authentication methods, including session-based authentication, token-based authentication (API authentication), and socialite authentication (integration with popular social media platforms for authentication).
Q) Can I customize the login and registration process in Laravel?
A) Yes, you can customize the login and registration process in Laravel by modifying the generated views, routes, and controllers or by creating your own custom logic and views to meet your specific requirements.
Q) Are there any packages or libraries that can help streamline Laravel login authentication?
A) Yes, popular packages like Laravel Sanctum, Laravel Passport, Laravel Socialite, and Laravel Jetstream can streamline Laravel login authentication for various needs.
Q) Can I implement social login functionality (e.g., login with Google or Facebook) in Laravel?
A) Yes, you can easily implement social login functionality (e.g., Google or Facebook) in Laravel using the Laravel Socialite package. It provides a simple way to authenticate with OAuth providers and supports popular platforms like Google, Facebook, Twitter, GitHub, and more.
Q) What is the purpose of implementing API rate limiting in Laravel?
A) API rate limiting in Laravel controls request volume to prevent abuse, protect server resources, maintain fairness, and ensure optimal API performance.
Q) How can I set up API rate limiting in Laravel?
A) Follow the steps below to set up API rate limiting in Laravel:
- Open the app/Providers/RouteServiceProvider.php file.
- Locate the ‘api‘ route middleware group within the map() method.
- Add the throttle:rate_limit,expire middleware to the desired routes or middleware group within the ‘api‘ middleware group.
- Replace rate_limit with the maximum number of allowedrequests and expire with the duration in minutes or seconds during which the rate limit applies.
- Save the changes to the RouteServiceProvider.php file.
Q) Are there different methods or strategies for implementing API rate limiting in Laravel?
A) Yes, Laravel offers multiple methods and strategies for implementing API rate limiting. These include:
Utilizing the built-in throttle middleware.
Implementing custom rate limiting logic using middleware or custom middleware classes.
Employing external packages like “Laravel-Rate-Limiter” for advanced rate limiting capabilities.
Q) Are there any tools or packages available to simplify the implementation of API rate limiting in Laravel?
A) Yes, packages such as “Laravel-Rate-Limiter” and “Laravel-RateLimit” provide simplified API rate limiting with additional features and customization options.
Shahzeb Ahmed
Shahzeb is a Digital Marketer with a Software Engineering background, works as a Community Manager — PHP Community at Cloudways. He is growth ambitious and aims to learn & share information about PHP & Laravel Development through practice and experimentation. He loves to travel and explore new ideas whenever he finds time. Get in touch with him at [email protected]