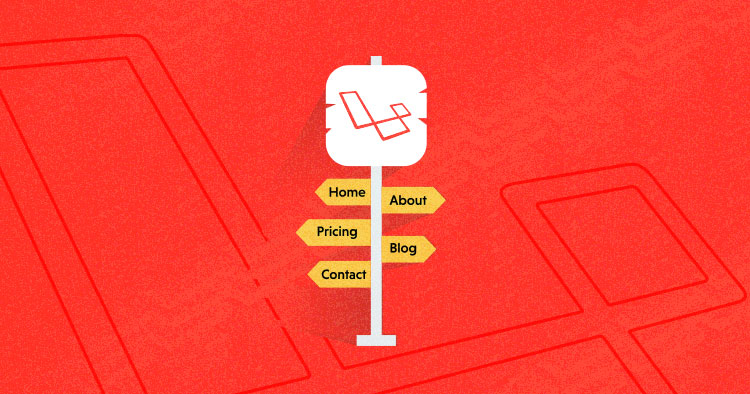
This article focuses on the concept of routing in Laravel, as previously, I had covered the basic introduction to installing Laravel.
Routing in Laravel allows users to route all their application demands to its appropriate controller. Most primary routes in Laravel acknowledge and accept a Uniform Asset Identifier together with a closure, giving a simple and expressive way of routing.
What Is a Route?
The route is a way of creating a request URL for your application. These URLs do not have to map to specific files on a website, and are both human readable and SEO friendly.
In Laravel, routes are created inside the routes folder. They are are created in the web.php
file for websites. And for APIs, they are created inside api.php
.
These routes are assigned to the net middleware group, highlighting the session state and CSRF security. The routes in routes/api.php are stateless and are assigned the API middleware group.
The default installation of Laravel comes with two routes, one for the web and the other for API. Here is how the route for web in web.php
looks like:
Route::get('/', function () { Â Â Â return view('welcome'); });
Stop Wasting Time on Servers
Cloudways handle server management for you so you can focus on creating great apps and keeping your clients happy.
What is a Route in Laravel?
All Laravel routes are defined in route files found within the routes directory. The application’s App\Providers\RouteServiceProvider automatically stacks these files. And the routes/web.php file defines the routes for your web interface.
You can define a route to this controller action, as:
Route::get(‘user/{id}’, ‘UserController@show’);
Route::resource: The Route::resource method can be a Restful Controller that produces all the basic routes required for an application and is dealt via controller class.
When a request matches the specified route URI, the show method on the App\Http\ControllersUserController lesson is invoked, passing the route parameters to the method.
For resources, you have to do two things on the Laravel application. Firstly, you must create a resource route on Laravel that provides insert, update, view, and delete routes. Secondly, create a resource controller that provides a method for insert, update, view, and delete.
The default installation of Laravel comes with two routes: one for the web and the other for API. Here is whatthe route for web in web.php looks like:
Route::get(‘/’, function () {
return view(‘welcome’);
});
Laravel Middleware acts as a bridge between the request and the reaction. It can be a sort of filtering component.
Laravel joins a middleware that confirms whether or not the client’s application is verified. In case the client is confirmed, it redirects to the home page or a login page.
Stop Wasting Time on Servers
The above code defines a route for the homepage. Every time this route receives a get request for /, It will return the view welcome. Views are the frontend of the application and I will discuss the topic in an upcoming installment of this series.
All Laravel routes are defined in your route files, which are found within the routes directory. Your application’s AppProvidersRouteServiceProvider consequently stacks these records. The routes/web.php record defines routes that are for your web interface.
The structure of the route is very simple. Open the appropriate file (either `web.php` or `api.php`) and start the code with `Route::`Â This is followed by the request you want to assign to that specific route and then comes the function that will be executed as a result of the request.
Laravel offers the following route methods:
- get
- post
- put
- delete
- patch
- options
Routes are defined in Laravel within the Route class with an HTTP verb, the route to reply to, and closure, or a controller strategy.
How to Create Routes in Laravel
Let’s see how you can create your own routes in Laravel.
Note: All the post, put, and delete requests require the CSRF token to be sent along with the request. Otherwise, the request will be rejected. Learn more about CSRF protection in Laravel official docs.
A Basic GET Route
I will now create a basic route that will print the table of 2.
Route::get('/table', function () { Â Â Â for($i =1; $i <= 10 ; $i++){ Â Â Â Â Â Â Â echo "$i * 2 = ". $i*2 ."<br>"; Â Â Â } Â Â });
In the above code, I created a GET request route for /table
, Â which will print the table of 2 on the screen.
Now, what if I want the user to decide the number for which the route will print the table. Here is the code for such a route:
Route::get('/table/{number}', function ($number) { Â Â Â for($i =1; $i <= 10 ; $i++){ Â Â Â Â Â Â Â echo "$i * $number = ". $i* $number ."<br>"; Â Â Â } Â Â });
I have defined a route parameter in the above code and passed it to the route function. Now, whenever a user route to /table
and add a number to the request (for instance, /table/3
), the function will print the table of that number to the screen.
Right now, I have created two separate routes (one that outputs 2’stable and the other that displays table for a user-defined number).
But is there any way of combining both functionalities in a single route?
Yes, there is one. Laravel offers the functionality of Optional Parameters that lets you add optional parameters by adding ? after the optional parameter and the default value. Let’s do this
Route::get('/table/{number?}', function ($number = 2) { Â Â Â for($i =1; $i <= 10 ; $i++){ Â Â Â Â Â Â Â echo "$i * $number = ". $i* $number ."<br>"; Â Â Â } Â Â });
In the above code we have made our route parameter, making the number optional, so if a user routes `/table` then it will generate 2’s table by default and if a user routes to `/table/{number}` then the table of that number will be printed.
Regular Expressions Constraints For Route Parameters
Above, we have created a route for table, but how do we ensure if the route parameter is actually a number to avoid errors while table generation?
In Laravel, you can define a constraint for your route parameter by using the `where` method on route instance. The `where` method takes parameter name and a regular expression rule for that parameter.
Let’s now define a constraint for our `{number}` parameter to assure that only a number is passed to the function.
Route::get('/table/{number?}', function ($number = 2) { Â Â Â for($i =1; $i <= 10 ; $i++){ Â Â Â Â Â Â Â echo "$i * $number = ". $i* $number ."<br>"; Â Â Â } Â Â })->where('number', '[0-9]+');
In the above code snippet, I defined a regular expression for our route’s number. Now if a user try to route to /table/no, a NotFoundHttpException will be displayed.
Laravel Routing with Controller Function
In Laravel, you can define a Controller method to a route. A controller method performs all the defined actions whenever a user comes to the route.
Use the following code snippet to assign a controller method to a route:
Route::get('/home', 'YourController@functionname');
The code starts with `Route::` and then defines the request method for the route. Next, define your route and the Controller along with the method by adding the @ symbol before the method’s name.
Naming Your Route
In Laravel, you can define name to your route. This name is often very useful in various scenarios. For example, if you want to redirect a user from one route to another, you do not need to define the full redirect URL. Instead, you can just give its name, and the code will work! You can define the route’s name by using the `name` method in the route instance.
Route::get('/table/{number?}', function ($number = 2) {    for($i =1; $i <= 10 ; $i++){        echo "$i * $number = ". $i* $number ."<br>";    }   })->where('number', '[0-9]+')->name(‘table’);
Now, I could re-generate the URL for this route through:
$url = route('table');
Similarly, for redirecting to this URL, the proper syntax would be:
return redirect()->route('table');
Conclusion
In this installment of the series, I discussed routing in Laravel 5.5. I have also covered related concepts regarding routes, like regular expressions and naming the routes. You can also refer to the official Laravel routing documentation to learn more about routing.
Shahzeb Ahmed
Shahzeb is a Digital Marketer with a Software Engineering background, works as a Community Manager — PHP Community at Cloudways. He is growth ambitious and aims to learn & share information about PHP & Laravel Development through practice and experimentation. He loves to travel and explore new ideas whenever he finds time. Get in touch with him at [email protected]