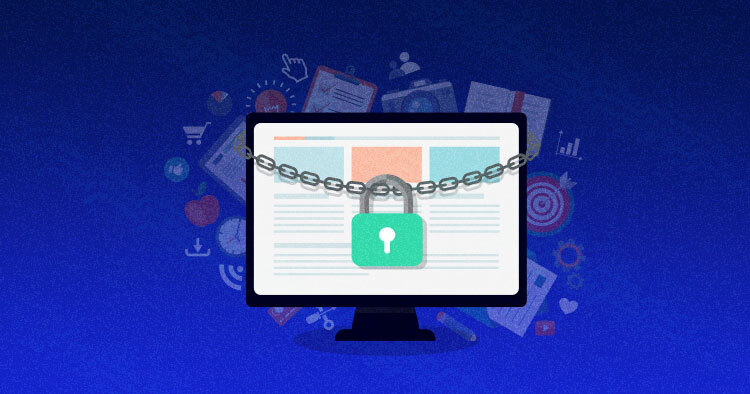
PHP encryption is important to the privacy and safety of your data. In practical terms, PHP encryption uses algorithms (sometimes called hashing algorithms) to translate the “clear” data into encrypted text that requires very specific decryption processes to “decode” the data back to the clean version.
These mathematics-based algorithms scramble plain text into gibberish ciphertext that can only be decoded by the intended recipient who possesses the “key”. A common example is the HTTPS encryption that protects client-server communication.
Stop Wasting Time on Servers
Cloudways handle server management for you so you can focus on creating great apps and keeping your clients happy.
Why encryption is important for data protection?
Encryption is a basic, but important component of any application because it allows you to securely protect data that you don’t want anyone else to access. This has attained newer significance in the age where personal or business information is normally stored in “cloud” servers.
For businesses, a lack of proper encryption could result in high damages because a leak of classified business data could benefit competitors. A leak of customers’ information is not just a PR nightmare; it could lead to lawsuits and business dissolution.
This is why encryption, especially tough-to-break password encryption, is one of the basic PHP security features customers expect from any PHP application.
The process is used to encrypt passwords:
- Create a unique encryption key (DEK)
- Scramble the information utilizing unique key encryption.
- Move the unique encryption key (DEK) to Cloud KMS for encryption, which returns the KEK.
- Save the encrypted data and key (KEK) along with each other.
- Take down the generated key (DEK)
How does Mysql Encrypt PHP Passwords?
MySQL doesn’t store passwords as plaintext but rather as a hashed value calculated by the Password() function. Using the Password() function to encrypt passwords is acceptable, but you can use more potent encryption methods. PHP MySQL password encryption docs explicitly state that Password() should only be used to manage passwords for MySQL internal accounts. Whenever we create a new user account using the CREATE USER command, MySQL takes the IDENTIFIED BY value and runs it through the Password() function behind the scenes. For that purpose, they recommend going with something a little more potent like SHA1 or Hash() and a random salt per Password to generate their rainbow tables based on the common salt. That ought to be strong for most purposes.
Methods of PHP Encryption
At the moment, developers can opt for several encryption methods, common being hashing, secret key encryption, and envelope encryption method. Every encryption method comes with multiple algorithms or ciphering (each with its own strengths and weaknesses).
Hashing
Applications allow users to enter personal information that is then saved in the database. Now developers need to make sure that this data remains unreadable.
Now imagine a scenario where a hacker forces their way to the databases and gains access to the user data. Because of hashing, this data would remain unreadable.
The hash produced by password_hash() is exceptionally secure. But you’ll be able to make it more grounded with two simple procedures: by expanding the Bcrypt cost, or by automatically updating the hashing algorithm
Here we are using a PHP function called password_hash which is a really strong one-way hashing algorithm we’re going to use bcrypt which is the algorithm that is built into the PHP language which gets updated as times goes on which make sure this method is always up to date so compare to other hash methods we used to have in past called md5 or state 256 this one is always up to date and should be used when you want to have passwords for some other kind of information inside a website.
Salt function is already included inside the password_hash, Salt is basically when we add a random string to our password before inserting it inside the database to make it even harder for people to figure out what password is.
This is the example of using hashing technique ‘bcrypt’.
Input:
<?php echo "Shahzeb Ahmed"; echo "<br>"; echo password_hash("Cloudways", PASSWORD_DEFAULT); ?>
Output:
Shahzeb Ahmed $2y$10$LZExuh/rXamd/X5yuDatn.L8ROlKBry3xidvWc.O19nx85.CrSrO.
You want to be able to see the password once we de-hash it because it is designed to be as safe as possible. This means that even YOU as the website owner should not be able to read other passwords.
Secret Key Encryption
Secret Key Encryption is also called Symmetric encryption, The Secret Key Encryption of the PHP uses just one key, called a shared secret, for both encrypting and decrypting.
To encrypt the data, Here one same key is used by the sender (for encryption) and the receiver (for decryption). So the key is shared. This key should be kept secret from all other parties to ensure privacy.
This is a simple, easy-to-use method of encryption, but there is one problem with it: The key must be shared between the sender and the recipient of the data, Also it is not preferred to use a symmetric encryption method where it uses a public network for sharing the key. Otherwise, if a third party intercepts the key during the exchange, an unauthorized person can easily decrypt the data.
Envelope encryption
Enveloping encryption is the idea that you put the data in a virtual envelope by encrypting it, lock it for one or more receivers (encrypting the data encryption key, for instance using RSA), and then put the receiver address, type of lock (the algorithm) and required key identifier on the outside.
We can use Cloud KMS service that can use a key to encrypt, decrypt, or sign data such as secrets for storage. which is actually provided by the Google Cloud Key Management Service.
How to encrypt and decrypt passwords using PHP?
The easiest way to protect passwords in PHP is to use the password_hash and verify functions.
The below example shows the method of using the password_hash() method:
Input:
<?php // The unencrypted password to be hashed $unencrypted_password = "Cloudways@123"; // The hash of the password can be saved in the database $hash = password_hash($unencrypted_password, PASSWORD_DEFAULT); // Print the generated hash code echo "Generated hash code: ".$hash; ?>
Output:
Generated hash code: 2y10D6pwJsA5KKdUs7tlrIC3z.we7QZR58wx9gEiuLlQ/dr7E/Rtnj9Ce
The decryption of the password: To decrypt a password hash and retrieve the original string, we use the password_verify() function.
Syntax:
bool password_verify(string $password, string $hash)
Verifies that the given hash matches the given password.
The password_verify() function verifies that the given hash matches the given password, generated by the password_hash() function. It returns true if the password and hash match, or false otherwise.
<?php // Plaintext password entered by the user $unencrypted_password = "Cloudways@123"; // The hashed password can be retrieved from database $hash ="2y10D6pwJsA5KKdUs7tlrIC3z.we7QZR58wx9gEiuLlQ/dr7E/Rtnj9Ce"; // Verify the hash code against the unencrypted password entered $verify = password_verify($unencrypted_password, $hash); // Print the result depending if they match if ($verify) { echo 'Correct Password!'; } else { echo 'Password is Incorrect'; } ?>
Output:
Correct Password!
Conclusion
I hope you learned the importance of PHP Encryption and along with the various types of PHP Encryption methods with examples. You can’t replace an SSL certificate with a hashed password on the user side and it will be actually unnecessary to hash it in the first place. Hashing passwords only make sense on the server machine.
What is the best way to encrypt password in PHP?
PHP encompasses a hash algorithm to encrypt the password. For the most part it is used in functions for password encrypting are crypt(), password_hash() and md5().
Shahzeb Ahmed
Shahzeb is a Digital Marketer with a Software Engineering background, works as a Community Manager — PHP Community at Cloudways. He is growth ambitious and aims to learn & share information about PHP & Laravel Development through practice and experimentation. He loves to travel and explore new ideas whenever he finds time. Get in touch with him at [email protected]