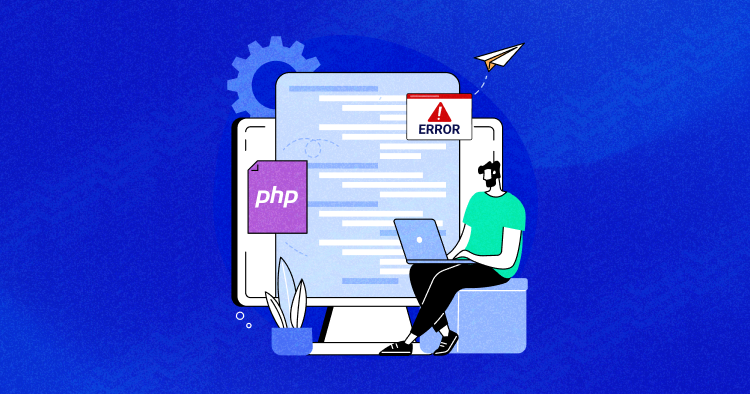
PHP offers strong methods to work with PHP error logs, which you can log manually and automate. Also, we have some third-party tools built by the open-source community to handle the PHP error logging process.
The most important thing is when and how to log errors. You can log PHP errors at your will when working in the dev mode. Either create a PHP error log file or save them as notifications on different channels, as you get the convenience of logging errors per your requirements.
This guide focuses on the basics of logs in PHP, their configuration, and where you can find them. Also, this guide details how logging helps you be more compelling when troubleshooting and monitoring your PHP applications.
When working in production mode, you must create a smooth workflow to enable PHP error logging so the users don’t face any glitches during runtime execution.
Stop Wasting Time on Servers
Cloudways handle server management for you so you can focus on creating great apps and keeping your clients happy.
Different Types of PHP Errors
PHP errors occur when something is off-base within the code. They can be as complex as calling an incorrect variable or as simple as missing a semicolon. You must understand the kind of errors you face to solve them effectively. So, let’s find out more about common PHP errors.
Warning Error
PHP warning errors alert about a problem that may cause a more critical error in the long run. Warning errors do not break down the code execution and commonly occur when using a file path that doesn’t exist.
For example, if this error pops up, you must check the file name in the code or directory, as the script may not find it due to the syntax error.
For instance:
<?php echo "Warning error"'; include ("external_file.php"); ? >
There is no file named “external_file,” so the output will display an error message box, and the execution will not break down.
Notice Error
Notice errors are minor; like warning errors, they don’t halt code execution. These errors can confuse the system in analyzing if there’s an actual mistake or if it’s just the standard code. Notice errors usually occur when the script needs access to an undefined variable.
Syntax Error
A syntax error is often caused by parsed, misused, or missing symbols. When the compiler catches the error, it terminates the script.
Parse/syntax errors are caused by:
- Unclosed brackets or quotes
- Missing or extra semicolons or parentheses
- Misspellings
Here’s an example of a parse error message:
PHP Parse error: syntax error, unexpected ‘5’ (L_NAME), expecting ‘)’ in /home/u802426761/domains/username/public_html/wp-config.php on line 32
Fatal Error
A fatal error happens when the code calls the function, but the function itself isn’t characterized. Unlike other PHP errors, a fatal error breaks down the execution and sometimes crashes the application as well. There are three types of fatal errors:
- A startup fatal error happens when the framework can’t run the code due to a mistake during installation.
- A compile-time fatal error occurs when the developer uses an undefined function, class, or non-existent variable or data.
- Runtime fatal error is similar to a compile-time fatal error, but happens during the program execution.
Here’s an example of a PHP fatal error:
PHP Fatal error: Call to undefined function get_header() in /var/www/username/public/blog/wp-content/themes/theme/index.php on line 37
Another reason for a fatal error is exceeding the execution time:
Fatal error: Maximum execution time of 30 seconds exceeded in /home/username/domains/domain.com/public_html/wp-includes/class-phpmailer.php on line 737
Getting Started with PHP Error Logging
PHP is the most widely used programming language for developing web applications. According to the BuiltWith insights, today almost.websites are using PHP as their backend language, which makes it around 75% of all the websites in the world. These stats clearly show that PHP still has a solid market share in the programming world. When you start developing an application in PHP, you use a few commands like print_r()
,var_dump()
to debug errors and log on to the browser. But, that is not the safest way while working in production mode. In dev mode, you can do it, but have to disable it when initiating the migration. Hence, in dev mode, you can easily log errors in PHP with error_log()
function, which sends an error message to the defined error handling routines. Let’s suppose you are connecting MySQL database with PHP and if it fails to connect with it, you can log errors in PHP like this:
<?php // Send error message to the server log if error connecting to the database if (!mysqli_connect("localhost","bad_user","bad_password","my_db")) { Â Â Â error_log("Failed to connect to database!", 0); } // Send email to administrator if we run out of FOO if (!($foo = allocate_new_foo())) { Â Â Â error_log("Oh no! We are out of FOOs!", 1, "[email protected]"); } ?>
Enable Error Logging in php.ini
To log errors in PHP, open the php.ini file and uncomment/add the following lines of code.
error_reporting = E_ALL & ~E_NOTICE error_reporting = E_ALL & ~E_NOTICE | E_STRICT error_reporting = E_COMPILE_ERROR|E_RECOVERABLE_ERROR|E_ER… _ERROR error_reporting = E_ALL & ~E_NOTICE
If you want to enable PHP error logging in individual files, add this code at the top of the PHP file.
ini_set('display_errors', 1); ini_set('display_startup_errors', 1); error_reporting(E_ALL);
Now, you must enable only one statement to parse the log errors in the php.ini file:
display_errors = on.
Now, you can easily see logged errors in your browser. Some of the additional commands you can write for PHP error logging include:
// Turn off all error reporting error_reporting(0); // Report simple running errors error_reporting(E_ERROR | E_WARNING | E_PARSE); // Reporting E_NOTICE can be good too (to report uninitialized variables or catch variable name misspellings ...) error_reporting(E_ERROR | E_WARNING | E_PARSE | E_NOTICE); // Report all errors except E_NOTICE error_reporting(E_ALL & ~E_NOTICE); // Report all PHP errors (see changelog) error_reporting(E_ALL); // Report all PHP errors error_reporting(-1); // Same as error_reporting(E_ALL); ini_set('error_reporting', E_ALL);
How Do I View PHP Logging Error?
You can check the PHP error log only when it is enabled; in most cases, it is disabled by default. So, before changing code and the configuration, check the PHP info to ensure that PHP logging is enabled on your PHP hosting account.
Method 1: Using the .htaccess File
You can enable the PHP error log by editing the .htaccess file. Find the .htaccess file in the site’s root directory using the file manager or an FTP client. However, go to the control panel’s settings or FTP client to show hidden files if the file is hidden.
Once you’ve found the .htaccess file, follow the steps below:
- Open the .htaccess file and insert the following code:
php_flag log_errors on
php_value error_reporting 32767
php_value error_log “error_log.txt - Create a file titled error_log.txt in the public_html directory
- Save and close the file
- All PHP error logs will be reported in the error_log.txt file
Now the PHP error logging is enabled, verify using the PHP info again, and the Local Value should be on. Don’t worry if the Master Value is still off, as the local value will override it.
If the website experiences any trouble, open the error_log.txt, and you’ll see the PHP error logs.
Method 2: Using php.ini File
The third method to enable the PHP error log is editing the php.ini file. It contains the default web server configuration to run PHP applications.
You can find the php.ini file by accessing the PHP info. Once you open it, press CTRL + F for Windows or Command + F for MacOS to open the search bar and look for the Loaded Configuration File section.
Another method to find the configuration file is using the command line.
Connect to the web server by using an SSH client and execute the following command:
php -i | grep php.ini
It should print output like this:
That said, here are the common directories for php.ini file depending on the operating system:
- CLI – /etc/php/7.4/cli/php.ini. Note that changing the PHP configuration in CLI won’t affect the web server.
- Apache – /etc/php/7.4/apache2/php.ini.
- Nginx or Apache with PHP-FPM – /etc/php/7.4/fpm/php.ini.
Copy PHP Error Logs to File
The above-defined practices work well when working in the dev environment. But, when you take your website live and start working in production mode, you must hide the errors from the on-screen display and log them within a backend file.
You can save these at a specific PHP error logs location whose URL is already described in the php.ini file.
PHP stores error logs in /var/log/apache2,if it uses an apache2 module. Shared hosts tend to store PHP error log files in your root directory /log subfolder. But…if you have access to the php.ini file you can do this by:
error_log = /var/log/php-scripts.log
If you’re using cPanel, the master log file, what you’re probably looking for is stored (by default) at:
/usr/local/apache/logs/error_log
If all else fails, you can check the PHP error logs location by using:
<?php phpinfo(); ?>
Error logging in PHP Frameworks
The above steps I’ve explained are for core PHP development. PHP has a variety of Model View Architecture (MVC) and micro-frameworks which have their methods on top of the core functions. Some of the best PHP MVC frameworks to build advanced web applications which include: Laravel, CodeIgniter, and Symfony.
Error Logging In Laravel
When you set up a new Laravel project, error logging and exception handling come pre-configured in it. Laravel has a separate class App\Exceptions\Handler which takes care of all these issues. For logging Laravel errors, it utilizes the Monolog library which supports a variety of log handlers. Laravel configures several of these handlers for you, allowing you to choose between a single PHP error log file, rotating log files, and writing error information to the system log.
In the config/app.php file, you can configure the PHPÂ option to log the errors on the display of the user. The value of this option can be set in the .env file under property APP_DEBUG which is an environment variable, thus setting up in the app.php file. When developing locally, the value can be set to true, and after migrating to production, it must be set to false. Otherwise, the security concern is always there as it will show error on the browser screen.
You, being the developer, can save Laravel log information on a single file, daily file, Syslog, and the errorlog. To configure these options for Laravel error logging, you must open the app.php file and edit the log option. For instance, if you want to set up daily logging for errors, you will do the following:
'log' => 'daily'
Monolog can log errors with different security warnings. By default, it adds all the errors in storage, but you can identify them as error, emergency, alert, critical, and warning. To do that, add ‘log_level‘ property in options like this:
'log_level' => env('APP_LOG_LEVEL', 'error'),
The log file is present in storage/logs. You can also log errors using log facades.
<?php namespace App\Http\Controllers; use App\User; use Illuminate\Support\Facades\Log; use App\Http\Controllers\Controller; class UserController extends Controller {    /**     * Show the profile for the given user.     *     * @param  int $id     * @return Response     */    public function showProfile($id)    {        Log::info('Showing user profile for user: '.$id);        return view('user.profile', ['user' => User::findOrFail($id)]);    } } The logger provides eight different logging levels, including: Log::emergency($message); Log::alert($message); Log::critical($message); Log::error($message); Log::warning($message); Log::notice($message); Log::info($message); Log::debug($message); Error Logging In Symfony
Symfony comes with a default logger which you can inject into the controller showing different warning levels. By default, log entries are written in the var/log/dev.log file when you’re in the var/log/prod.log
use Psr\Log\LoggerInterface; public function index(LoggerInterface $logger) {    $logger->info('I just got the logger');    $logger->error('An error occurred');    $logger->critical('I left the oven on!', [        // include extra "context" info in your logs        'cause' => 'in_hurry',    ]);    // ... }
Since Symfony is the parent framework for Laravel, the warning levels of the framework are also the same.
Symfony also uses Monolog for error logging and pre-configures some basic handlers in the default monolog.yaml. Below is the example which uses syslogs to write logs on the file:
// config/packages/prod/monolog.php $container->loadFromExtension('monolog', [ Â Â Â 'handlers' => [ Â Â Â Â Â Â Â 'file_log' => [ Â Â Â Â Â Â Â Â Â Â Â 'type' => 'stream', Â Â Â Â Â Â Â Â Â Â Â 'path' => '%kernel.logs_dir%/%kernel.environment%.log', Â Â Â Â Â Â Â Â Â Â Â 'level' => 'debug', Â Â Â Â Â Â Â ], Â Â Â Â Â Â Â 'syslog_handler' => [ Â Â Â Â Â Â Â Â Â Â Â 'type' => 'syslog', Â Â Â Â Â Â Â Â Â Â Â 'level' => 'error', Â Â Â Â Â Â Â ], Â Â Â ], ]);
Automating PHP Error Logging Process
You can do the whole work of error logging manually, but when you are working in multiple teams and larger projects, you must set up an automated workflow that can log errors in third-party services like Sentry, Slack, Blackfire, etc.
These tools can provide you a better understanding of errors and their solutions. You can also set up Slack channels to send quick notifications to the teams about any runtime incidents.
You can see this nice example in Symfony which also works similarly.
Assume you have already set up the Slack channel and webhooks as:
monolog:    handlers:        main:            type:  fingers_crossed            action_level: error            handler:  nested        nested:            type: stream            path: "%kernel.logs_dir%/%kernel.environment%.log"            level: debug        console:            type: console        slack:            type: slack            token: xxxx-xxxxxxxxxxx-xxxxxxxxx-xxxxxxxxxx-xxxxxx            channel: "#name-of-channel"            bot_name: ChooseName            icon_emoji: :ghost:            level: critical
The emojis are also set up in the above example, have you seen that? 😀 This shows how you can send Slack notifications to any particular channel as per your needs.
How PHP Errors Are Logged on Cloudways
When you create an account on Cloudways web hosting for PHP and launch the PHP server with the application, you can see the log folder at the root directory which takes care of the server logs. You can find both Apache and Nginx logs there, which help you to identify the potential error logs. Since Cloudways does not allow users to edit php.ini file (you can ask a live support agent to do that for you); but if you carefully see the PHP server settings, you can customize most of the error_reporting and logging settings in the platform.
Of course, you also have access to PHP-FPM where you can add php.ini rules to log PHP errors.
Host PHP Websites with Ease [Starts at $10 Credit]
- Free Staging
- Free backup
- PHP 8.0
- Unlimited Websites

Final Words
PHP error log helps you troubleshoot problems on a website by logging the error details, including the PHP file and code line that needs fixation.
This article has demonstrated how logging errors in PHP are configured in the php.ini file. Further, it discusses the right way to log errors and how to enable and automate PHP error logging, keeping the dev work on track.
If you find this article helpful and want to share your views about the topic, feel free to write down your suggestions in the comments below.
Q: Which PHP error logging library is best to use?
A: Monolog is one of the best PHP error logging libraries available in the market. It provides advanced logging operations for different handlers including database, browser console, chats solutions, and others.
Q: What to do if the PHP error log is not working?
A: First, set the log_errors = on in the php.ini file, it can help if your PHP error logging is not working.
If it still doesn’t work, make sure that the error_log directive is set and the directory in it must be writable by the user that Apache is running under.