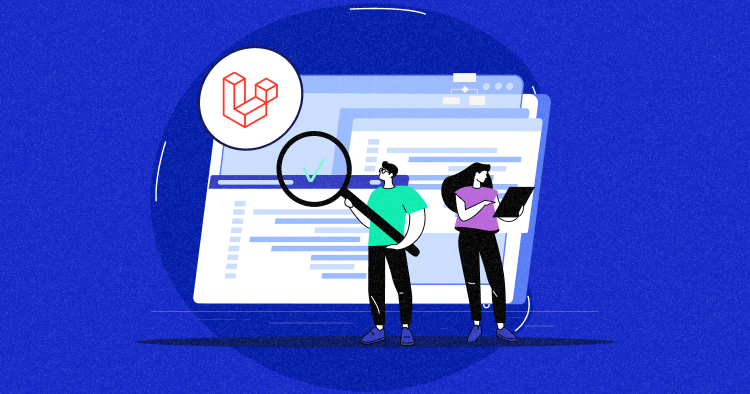
Inertia serves as a game-changer in the realm of Laravel development, offering a more integrated and streamlined approach to building modern web applications.
Inertia with Laravel offers a familiar workflow for developers already accustomed to working with Laravel. It significantly reduces the learning curve typically associated with transitioning to a completely separate frontend framework.
This ease of integration allows developers to leverage their existing Laravel skills and benefit from Inertia’s ability to modernize the frontend experience without requiring a complete application re-architecture.
Using Inertia with Laravel offers many benefits, from unified stack advantages to improved efficiency, creating a more seamless and developer-friendly experience.
In this blog post, we’ll delve into the reasons for using Inertia with Laravel, explore its functionality, and guide you through the process of getting started with Laravel Inertia on Cloudways.
Why Use Inertia With Laravel?
Inertia.js is a powerful tool that seamlessly blends modern frontend capabilities with server-side frameworks like Laravel. Its integration with Laravel offers a substantial enhancement to traditional server-rendered web applications.
The primary advantage lies in its ability to create more dynamic and interactive user interfaces while preserving the robust backend features that Laravel is renowned for.
The shared code structure between frontend and backend operations not only enhances developer productivity but also contributes to better maintainability and scalability of the application.
What Problem Does Laravel Inertia Solve?
Inertia with Laravel streamline the development process by facilitating a single-page application (SPA) feel without fully transitioning to a full-fledged SPA architecture. This allows developers to build reactive, dynamic interfaces using modern frontend frameworks like Vue.js or React while benefiting from the server-side rendering capabilities of Laravel.
Moreover, Inertia simplifies the data flow between the frontend and backend by using Laravel controllers to handle both frontend and backend logic. This cohesion streamlines the development process, eliminating the need to create separate API endpoints and ensuring a more coherent codebase.
How Does Inertia Work?
Inertia.js fundamentally transforms the way frontend and backend interact within a web application. At its core, Inertia provides a modern frontend experience while maintaining the traditional server-driven Laravel backend architecture. It achieves this by creating a bridge that connects the backend and frontend seamlessly.
When a user interacts with the frontend, instead of making traditional full-page requests, Inertia utilizes XHR (XMLHttpRequest) or Fetch API to communicate with the backend. This communication transmits only the essential data needed for the specific action.
Working Mechanisms
- On a user click, the front end sends a request to the server using this mechanism, triggering the relevant Laravel controller method.
- The controller processes the request and returns an Inertia response that includes the necessary data for updating the page without a full reload.
- The Inertia client on the front end receives this response, uses the data to update the page content, and seamlessly modifies the user interface based on the received information.
In simple terms, this is how Inertia works.
Why Use With Single Page Application (SPA)?
Inertia with a Single Page Application (SPA) architecture offers a potent combination that leverages the strengths of both approaches.
SPAs, by design, offer a highly responsive, fluid user experience by loading a single HTML page and then dynamically updating its content as users interact with the application.
- Enhanced User Experience: SPAs excel in providing seamless, app-like experiences, reducing page reloads, and delivering content dynamically. Inertia complements this by allowing SPAs to maintain this high level of interactivity while benefiting from Laravel’s backend structure.
- Simplified Development: Combining Inertia with a SPA reduces the complexity of managing frontend routing, state, and API integrations. Inertia’s approach allows developers to harness the power of SPAs while keeping the backend and frontend communication straightforward.
Consistent Development Workflow: Inertia maintains a workflow that aligns with Laravel’s structure, making it easier for developers already familiar with Laravel to transition to a more modern, SPA-based approach without learning entirely new front-end technologies.
Experience the Perfect Synergy of Laravel Inertia and Cloudways Hosting
Launch, scale, and optimize your applications seamlessly. Witness the unparalleled performance of your Laravel Inertia projects with Cloudways!
Getting Started on Laravel Inertia With Cloudways
To understand Inertia and how to integrate it with Laravel, we’re going to build a blog web app named CW Blog using the most powerful combo, Laravel for the backend, Vue.js for the JavaScript frontend, and Tailwind CSS for styling.
Prerequisites
To get the most out of this tutorial, you should be familiar with the following:
- Laravel basics (installation, database, database migrations, Eloquent Models, controllers, and routing)
- Vue.js basics (installation, structure, and forms)
Step 1: Install Core Elements
- We’re gonna start off by creating a single page application.
- Create a Blade component for viewing the blog’s homepage and a single article on the blog as “/resources/views/index.blade.php”.
<!DOCTYPE html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <title>CW Blog</title> </head> <body> <header> <h1>CW Blog</h1> </header> <main> <h2>Read our latest articles</h2> <section> <article> <div> <img src="/images/CW-logo.png" alt="Article thumbnail" /> </div> <h3>Title for the blog</h3> <p> </p> <a href="#">Read more</a> </article> </section> </main> <footer> <h2>Join our Newsletter</h2> <input type="email" /> </footer> </body> </html>
Step 2: Setting up Blade File
- Now its time to create a new file with a .blade.php extension.
- To do this, in your Laravel project blog/resources/views, navigate to the “/resources/views/show.blade.php“:
<!DOCTYPE html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <title>CW Blog</title> </head> <body> <main> <article> <div> <img src="/images/CW-logo.png" alt="Article thumbnail" /> </div> <h1>Title for the blog</h1> <p>Article content goes here</p> </article> </main> <footer> <h2>Join our Newsletter</h2> <input type="email" /> </footer> </body> </html>
- Create a new MySQL local database named CW_blog and connect it to your project:”.env“:
DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=CW_blog DB_USERNAME=root DB_PASSWORD=
- Run database model, migrations, and factories in your laravel application :”app/Models/Article.php“:
<?php namespace AppModels; use Illuminate\Database\Eloquent\Factories\HasFactory; use Illuminate\Database\Eloquent\Model; class Article extends Model { use HasFactory; protected $fillable = ['title', 'excerpt', 'body']; }
- Run database migration and export the demo articles to your database to “database/migrations/create_articles_table.php“:
<?php use Illuminate\Database\Migrations\Migration; use Illuminate\Database\Schema\Blueprint; use Illuminate\Support\Facades\Schema; return new class extends Migration { public function up() { Schema::create('articles', function (Blueprint $table) { $table->id(); $table->string('title'); $table->text('excerpt'); $table->text('body'); $table->timestamps(); }); } public function down() { Schema::dropIfExists('articles'); } };
- Run the following code and create a new class ArticleFactory that will store our demo articles in our database.
<?php namespace DatabaseFactories; use Illuminate\Database\Eloquent\Factories\Factory; class ArticleFactory extends Factory { public function definition() { return [ 'title' => $this->faker->sentence(6), 'excerpt' => $this->faker->paragraph(4), 'body' => $this->faker->paragraph(15), ]; } }
That’s all we need to get started! Let’s get down to business and introduce Inertia.js to our project.
Install Inertia
The Inertia installation process is divided into two major phases: server-side (Laravel) and client-side (VueJs).
The official installation guide in the Inertia documentation is a little outdated because Laravel 9 now uses Vite by default, but we’ll go through that as well.
Step 1: Setting Up Server-Side
The first thing we need to do is use the terminal to install the Inertia server-side adapters using Composer. To use InertiaJS with Laravel, you need to install the required package by running ‘composer require inertiajs inertia-laravel’.
- Now, set up a root template file in Blade, which will load your CSS and JS files and the Inertia root to launch your JavaScript application. It’s crucial to activate Vite’s functionality in conjunction with Laravel 9 v9.31 by adding it to our tags in /resources/views/app.blade.php:
<!DOCTYPE html> <html lang="{{ str_replace('_', '-', app()->getLocale()) }}"> <head> <meta charset="utf-8" /> <meta name="viewport" content="width=device-width, initial-scale=1" /> <!-- Fetch project name dynamically --> <title inertia>{{ config('app.name', 'Laravel') }}</title> <!-- Scripts --> @vite('resources/js/app.js') @inertiaHead </head> <body class="font-sans antialiased"> @inertia </body> </html>
- Next, we will create HandleInertiaRequests middleware and publish it to our project.
- We can do that by firing the below terminal command within the root directory of our project:
php artisan inertia:middleware
- Once this is complete, head to “App/Http/Kernel.php” and register HandleInertiaRequests as the last item in your web middleware:
'web' => [ // ... App\Http\Middleware\HandleInertiaRequests::class, ],
Step 2: Setting up Client-Side
Next, we have to install our frontend Vue.js 3 dependencies in the same way as on the server side:
npm install @inertiajs/inertia @inertiajs/inertia-vue3
- Next, you need to pull in Vue.js 3:
npm install vue@next
- Update your primary JavaScript file to initialize Inertia.js with Vue.js 3, Vite, and Laravel:
import "./bootstrap"; import "../css/app.css"; import { createApp, h } from "vue"; import { createInertiaApp } from "@inertiajs/inertia-vue3"; import { resolvePageComponent } from "laravel-vite-plugin/inertia-helpers"; createInertiaApp({ title: (title) => `${title} - ${appName}`, resolve: (name) => resolvePageComponent( `./Pages/${name}.vue`, import.meta.glob("./Pages/**/*.vue") ), setup({ el, app, props, plugin }) { return createApp({ render: () => h(app, props) }) .use(plugin) .mount(el); }, });
- We use Laravel’s plugin resolvePageComponent and instruct it to resolve our components from the directory ./Pages/$name.vue.
- This is to save our Inertia components in this directory later in our project, and this plugin will assist us in automatically loading those components from the correct directory.
- All that is left is to install vitejs/plugin-vue:
npm i @vitejs/plugin-vue
- And update vite.config.js file:
import { defineConfig } from "vite"; import laravel from "laravel-vite-plugin"; import vue from "@vitejs/plugin-vue"; export default defineConfig({ plugins: [ laravel({ input: ["resources/css/app.css", "resources/js/app.js"], refresh: true, }), vue({ template: { transformAssetUrls: { base: null, includeAbsolute: false, }, }, }), ], });
- The final step is to install our dependencies and compile our files:
npm install npm run dev
That is it. You have a working Laravel 9 application with Vue.js 3 and Vite.
Creating Inertia Pages
- Now we are going to create a folder called “Pages” and move your files there.
- Then we will transform the blade component “.blade.php” to “.vue” while making the first letter of their names uppercase and turn its content into a standard Vue.js component.
- We will move all the tags along with the components as they are already included in the main root blade component.
<script setup> // </script> <template> <header> <h1>CW Blog</h1> </header> <main> <h2>Read our latest articles</h2> <section> <article> <div> <img src="/images/CW-logo.png" alt="Article thumbnail" /> </div> <h3>Title for the blog</h3> <p> Article Content Goes Here.</p> <a href="#">Read more</a> </article> </section> </main> <footer> <h2>Join our Newsletter</h2> <input type="email" /> </footer> </template>
Setting up show.vue
We’re now going to set up the “resources/js/Pages/Show.vue“ page, which is going to be the layout of our application.
<script setup> // </script> <template> <header> <h1>Welcome to CW Blog</h1> </header> <main> <article> <h1>Title for the blog</h1> <p>Article content goes here</p> </article> </main> <footer> <h2>Join our Newsletter</h2> <input type="email" /> </footer> </template>
Wrapping Components
- Create a folder named “Layouts” in “/resources/js.” In this folder, create a file named “CWLayout.vue.”
- The file will contain separate sections for the headers and footers, with a main section designed to accommodate extra components.
This file should look like this:
<script setup></script> <template> <header> <h1>CW Blog</h1> </header> <main> <slot /> </main> <footer> <h2>Join our Newsletter</h2> <input type="email" /> </footer> </template>
Creating Index.vue:
Now, we’ll import the new layout into our pages and wrap all the HTML content in it.
<script setup> import CWLayout from "../Layouts/CWLayout.vue"; </script> <template> <CWLayout> <section> <h2>Read our latest articles</h2> <article> <div> <img src="/images/CW-logo.png" alt="Article thumbnail" /> </div> <h3>Title for the blog</h3> <p>Article content goes here!</p> <a href="#">Read more</a> </article> </section> </CWLayout> </template>
Show.vue:
Run the following script to display the layout we’ve created in the previous script.
<script setup> import CWLayout from "../Layouts/CWLayout.vue"; </script> <template> <CWLayout> <article> <h1>Title for the blog</h1> <p>Article content goes here</p> </article> </CWLayout> </template>
Laravel Routes and Inertia Render
It’s time to seed some articles into our database that we created in the start of this tutorial.
<?php namespace Database\Seeders; use App\Models\Article; use Illuminate\Database\Seeder; class DatabaseSeeder extends Seeder { public function run() { Article::factory(10)->create(); } }
Run the following command to migrate your tables and seed the fake data from the factories:
php artisan migrate:fresh --seed
This will create 10 demo articles in the database, which we will need to pass to our view using Laravel routing.
Calling “routes/web.php“:
Since we’re using Inertia for views, Create Laravel Inertia route in “routes/web.php” and return the homepage view from “/resources/js/Pages/Index.vue“.
<?php use App\Models\Article; use Illuminate\Support\Facades\Route; use Inertia\Inertia; Route::get('/', function () { return Inertia::render('Index', [ 'articles' => Article::latest()->get() ]); })->name('home');
Go to the Index file and put the information you got as a prop. Then, use v-for to display the results in a loop. Within the script tags, set the received data as a prop.
Inertia needs to know what kind of information you want, like a list of articles.
<script setup> import CWLayout from "../Layouts/CWLayout.vue"; defineProps({ articles: Object, }); </script>
Let’s make the loop:
<template> <CWLayout> <h2>Read our latest articles</h2> <section> // Looping over articles <article v-for="article in articles":key="article.id"> <div> <img src="/images/CW-logo.png" alt="Article thumbnail" /> </div> <h3>{{article.title}}</h3> <p>{{article.excerpt}}</p> <a href="#">Read more</a> </article> </section> </CWLayout> </template>
Using Tailwind CSS With Inertia.js
- Since Tailwind is already installed in our project at the starting point, all we need to do is tell it to read our Inertia components. This may be accomplished by editing “tailwind.config.js” as follows:
/** @type {import('tailwindcss').Config} */ module.exports = { content: [ "./storage/framework/views/*.php", "./resources/views/**/*.blade.php", "./resources/js/**/*.vue", ], theme: { extend: {}, }, plugins: [], };
- Then make sure that we have imported our CSS file in “resources/js/app.js“:
import "../css/app.css";
- And now we’re ready to style our components in “resources/js/Pages/Index.vue“:
<script setup> import CWLayout from "../Layouts/CWLayout.vue"; defineProps({ articles: Object, }); </script> <template> <CWLayout> <h2 class="text-2xl font-bold py-10">Read our latest articles</h2> <section class="space-y-5 border-b-2 pb-10"> <article v-for="article in articles" :key="article.id" class="flex justify-center items-center shadow-md bg-white rounded-xl p-4 mx-auto max-w-3xl" > <img src="/images/CW-logo.png" class="w-32 h-32 rounded-xl object-cover" alt="" /> <div class="flex flex-col text-left justify-between pl-3 space-y-5"> <h3 class="text-xl font-semibold text-indigo-600 hover:text-indigo-800" > <a href="#">{{ article.title }}</a> </h3> <p> {{ article.excerpt }} </p> <a href="#" class="text-indigo-600 hover:text-indigo-800 w-fit self-end font-semibold" >Read more</a > </div> </article> </section> </CWLayout> </template>
- Add new style components in “resources/js/Layouts/CWLayout.vue“:
<script setup></script> <template> <header class="bg-gradient-to-r from-blue-700 via-indigo-700 to-blue-700 w-full text-center py-4" > <h1 class="text-white font-bold text-4xl">CW Blog</h1> </header> <main class="container mx-auto text-center"> <slot /> </main> <footer class="bg-gradient-to-b from-transparent to-gray-300 w-full text-center mt-5 py-10 mx-auto" > <h2 class="font-bold text-xl pb-5">Join our Newsletter</h2> <input class="rounded-xl w-80 h-12 px-3 py-2 shadow-md" type="email" placeholder="Write your email.." /> </footer> </template>
- There it is! You’ve got yourself a working SPA with 10 demo blogs in it, using Laravel Inertia
Optimize Your Laravel Inertia Experience With Cloudways Hosting!
Elevate your development process and unmatched performance for your applications. Join Cloudways now and unlock the full potential of your projects!
Laravel Inertia vs Traditional Frontend Development
Laravel Inertia presents a more integrated, streamlined approach by combining the backend and frontend technologies, reducing the complexities of managing separate codebases.
Here’s a detailed comparison table outlining the differences between Laravel Inertia and Traditional Frontend Development;
Aspect | Laravel Inertia | Traditional Frontend Development |
Technology Stack | Combines backend (Laravel) with Vue.js or React on frontend | Uses separate frontend frameworks (React, Vue.js) |
Development Speed | Speeds up development by reducing context switching | May require context-switch between backend and frontend frequently |
Rendering & Routing | Client-side rendering with server-managed routing | Server-side rendering with client-managed routing |
API Requests & Data Fetching | Simplified API requests with shareable Laravel routes | Manual handling of API requests and data fetching |
Template Engine Integration | Integrates with Blade for server-rendered templates | Relies on templating engines like Handlebars, EJS |
State Management | Simplifies state management using server-driven state | Manual state management with tools like Redux |
SEO & Page Load Performance | Enhanced SEO due to server-side rendering | SEO may require additional configurations |
Learning Curve | Reduced learning curve for developers familiar with Laravel | Requires proficiency in separate frontend frameworks |
Ecosystem & Community Support | Growing community support within Laravel ecosystem | Established communities around frontend frameworks |
Maintenance & Updates | Simplified maintenance with unified codebase | Updates might require synchronization of separate backend and frontend codes |
Code Simplicity & Readability | Promotes simpler code structure and better readability | Might involve complex code separation and management |
Testing | Easier backend/frontend integration for testing | Separate testing environments for frontend and backend |
Conclusion
Laravel Inertia tackles the age-old challenge of creating seamless, dynamic, and responsive web applications by simplifying the communication between the backend and frontend.
Traditional approaches often involve complex setups, juggling between different templating languages, and manually synchronizing data between the server and client.
Inertia’s seamless integration with Laravel offers advantages in terms of development speed, maintenance, and code simplicity.
Additionally, it simplifies state management and testing, leading to a more efficient and cohesive development experience compared to the traditional frontend development approach.
Q) Can I use Vue.js and React with Inertia?
No, Inertia.js is specifically designed to work with server-side frameworks like Laravel and Rails and is not directly compatible with Vue.js or React.
Q) Does Inertia replace Blade or other Laravel view engines?
No, Inertia does not replace Blade or other Laravel view engines; rather, it works in conjunction with them to manage client-side interactions.
Q) Does Inertia work well for SEO?
Inertia can compromise SEO because it relies on client-side rendering, which may not be as easily indexed by search engines compared to traditional server-side rendering.
Q) Can I migrate an existing Laravel project to use Inertia?
Yes, it’s possible to migrate an existing Laravel project to use Inertia by gradually integrating it into specific parts or pages of the application.