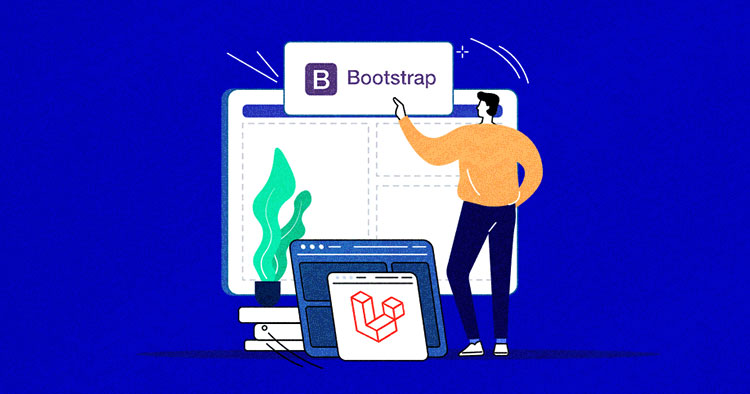
The task of merging front-end templates into Laravel often poses significant challenges for developers. It can be a complex, time-intensive process that may lead to disorganized code, thereby impacting the productivity of the development process.
Bootstrap, a widely-used front-end framework, offers a solution. When integrated with Laravel, it can streamline the development process, improve code organization, and enhance the visual appeal of your application.
This article presents a step-by-step guide to integrating a Bootstrap template with Laravel. It aims to empower you to build visually appealing and highly functional web applications with increased efficiency and reduced development time.
- Brief Overview of Laravel and Bootstrap
- Why Integrate Bootstrap With Laravel?
- System Requirements for Integration
- Setting up the Development Environment
- Understanding Bootstrap Templates
- Integrating Bootstrap With Laravel
- Installing Bootstrap Template in Laravel
- Downloading Dashboard With Laravel
- Best Bootstrap Templates for Laravel
Brief Overview of Laravel and Bootstrap
Laravel is a widely used framework for building web applications using PHP. It’s designed to make common tasks like routing, caching, and security easier for developers. Laravel provides ready-to-use tools for these tasks so developers can start coding immediately.
Bootstrap is a free tool for building responsive, mobile-first websites. It provides pre-made design templates for various elements like typography, forms, and buttons. Using Bootstrap can make your Laravel application look better and be more user-friendly.
Combining Laravel’s robust back-end capabilities with Bootstrap’s responsive front-end designs can make web development faster and more efficient, leading to a better web application.
Why Integrate Bootstrap With Laravel?
Integrating Bootstrap with Laravel can benefit web developers for several reasons:
- Efficiency: Laravel helps with back-end tasks, and Bootstrap saves time on front-end design. Using them together makes building web apps faster.
- Consistency: Bootstrap ensures your app looks good on all devices and platforms. This is great for apps that need to work on both desktop and mobile.
- Customizability: Bootstrap is flexible. You can change its designs to match your app’s look and feel.
- Community Support: Both Laravel and Bootstrap have large communities. You can find many resources, tutorials, and extra packages to help with your development.
- Future-Proof: Laravel and Bootstrap are regularly updated with the latest web development practices. Using them keeps your app modern and up-to-date.
System Requirements for Integration
To integrate Bootstrap with Laravel, you’ll need the following system requirements:
- Operating System: Laravel works on Windows, macOS, and Linux.
- PHP: You need PHP 7.3 or higher.
- Composer: This is used for managing dependencies in Laravel.
- Database: Laravel supports databases like MySQL, PostgreSQL, and SQL Server.
- Web Server: A web server like Apache or Nginx is needed to serve your Laravel application.
- Node.js and NPM: These are used to compile assets in Laravel.
- Bootstrap: You can get Bootstrap from its official website or use npm.
- Text Editor or IDE: You’ll need a tool like Visual Studio Code, Sublime Text, or PHPStorm to write your code.
Experience the Ease of Bootstrap Laravel Integration with Cloudways!
With support for all system requirements, from PHP 7.3 or higher, Composer, MySQL, Apache, Node.js, NPM, to Bootstrap, we’ve got you covered.
Setting up the Development Environment
Integrating Laravel with Bootstrap is a fantastic way to create web applications with a sleek and responsive user interface. To set up your development environment for this purpose, follow these steps:
Step 1: Install Laravel
Before integrating Bootstrap, you need to have Laravel installed. If you haven’t already, open your terminal and run the following command to create a new Laravel project:
composer create-project --prefer-dist laravel/laravel project-name
This command will create a new Laravel project in a directory named project-name.
Step 2: Set up Laravel Mix
Laravel Mix is a powerful tool for managing your application’s assets. In your Laravel project directory, open the webpack.mix.js file and configure it to compile your CSS and JavaScript assets.
mix.js('resources/js/app.js', 'public/js') .sass('resources/sass/app.scss', 'public/css');
Step 3: Install Bootstrap
You can add Bootstrap to your project using npm. Run the following command in your project directory. This will download Bootstrap and its dependencies into your project.
npm install bootstrap
Step 4: Import Bootstrap Styles and Scripts
Open your resources/sass/app.scss file and add the following line at the top to import Bootstrap’s styles.
@import '~bootstrap/dist/css/bootstrap.css';
Next, open your resources/js/bootstrap.js file and include Bootstrap’s JavaScript components:
require('bootstrap');
Step 5: Compile Assets
Now, run the following command to compile your assets using Laravel Mix. This command will compile your Sass and JavaScript files and copy them to the public directory.
npm run dev
Integrating Bootstrap With Laravel
The layout is a critical project component. Laravel addresses this by offering a Blade templating engine that produces sophisticated HTML-based designs and templates. All Laravel views constructed with Blade are housed in the resources/views directory.
Laravel, by default, uses NPM to install its frontend packages. Although Laravel does not enforce the use of specific JavaScript or CSS pre-processors, it provides a fundamental starting point with Bootstrap, React, and/or Vue that can be advantageous for numerous applications.
The integration of the Bootstrap template with Laravel is a straightforward procedure. It requires segmenting your HTML Bootstrap into smaller Blade template fragments. These fragments can then be utilized, extended, and/or included in the primary Blade file.
The Album Example For Bootstrap will be used to illustrate this process.
The code of your template is composed of various parts. To manage these effectively, we will create a layout where all the partial parts of the layout are kept separate.
Navigate to the resources/view folder and create a new folder named layouts. This folder will house the main layout and other files that will be included in the layout.
Next, create another folder and name it partials. This folder will contain partial files, such as the header and footer, which will be utilized by the layout file.
Finally, go to the layouts folder and create a file named mainlayout.blade.php. You can then add your desired code to this file. This file will serve as the primary layout for your application.
<!DOCTYPE html> <html lang="en"> <head> @include('layout.partials.head') </head> <body> @include('layout.partials.nav') @include('layout.partials.header') @yield('content') @include('layout.partials.footer') @include('layout.partials.footer-scripts') </body> </html>
This code generates a layout file that incorporates the contents of a specified file at a designated location within the HTML file using the
@include directive. The @yield directive is used to insert the specific content of a file that extends this layout.
Step 1: Create Partial Files
You’ll need to generate partial files that will be integrated into the main layout file. Navigate to the partials directory and establish a new file named head.blade.php. This file will contain the content that goes into the head section of the page.
<meta charset="utf-8"> <meta name="viewport" content="width=device-width, initial-scale=1, shrink-to-fit=no"> <meta name="description" content=""> <meta name="author" content=""> <title>Album example for Bootstrap</title> <!-- Bootstrap core CSS --> <link rel="stylesheet" href="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-alpha.6/css/bootstrap.min.css" integrity="sha384-rwoIResjU2yc3z8GV/NPeZWAv56rSmLldC3R/AZzGRnGxQQKnKkoFVhFQhNUIyJ" crossorigin="anonymous"> <!-- Custom styles for this template --> <link href="/css/album.css" rel="stylesheet">
Create a file nav.blade.php. This file will contain the code related to creating the navigation of the Bootstrap page.
<div class="collapse bg-inverse" id="navbarHeader"> <div class="container"> <div class="row"> <div class="col-sm-8 py-4"> <h4 class="text-white">About</h4> <p class="text-muted">Add some information about the album below, the author, or any other background context. Make it a few sentences long so folks can pick up some informative tidbits. Then, link them off to some social networking sites or contact information.</p> </div> <div class="col-sm-4 py-4"> <h4 class="text-white">Contact</h4> <ul class="list-unstyled"> <li><a href="#" class="text-white">Follow on Twitter</a></li> <li><a href="#" class="text-white">Like on Facebook</a></li> <li><a href="#" class="text-white">Email me</a></li> </ul> </div> </div> </div> </div> <div class="navbar navbar-inverse bg-inverse"> <div class="container d-flex justify-content-between"> <a href="#" class="navbar-brand">Album</a> <button class="navbar-toggler" type="button" data-toggle="collapse" data-target="#navbarHeader" aria-controls="navbarHeader" aria-expanded="false" aria-label="Toggle navigation"> <span class="navbar-toggler-icon"></span> </button> </div> </div>
Create a file header.blade.php.This file will contain the visible header of the Bootstrap page.
<section class="jumbotron text-center"> <div class="container"> <h1 class="jumbotron-heading">Album example</h1> <p class="lead text-muted">Something short and leading about the collection below—its contents, the creator, etc. Make it short and sweet, but not too short so folks don't simply skip over it entirely.</p> <p> <a href="#" class="btn btn-primary">Main call to action</a> <a href="#" class="btn btn-secondary">Secondary action</a> </p> </div> </section>
Create a file named footer.blade.php for the footer.
<footer class="text-muted"> <div class="container"> <p class="float-right"> <a href="#">Back to top</a> </p> <p>Album example is © Bootstrap, but please download and customize it for yourself!</p> <p>New to Bootstrap? <a href="../../">Visit the homepage</a> or read our <a href="../../getting-started/">getting started guide</a>.</p> </div> </footer>
Create a file named footer-scripts.blade.php containing the JS files that should be included at the bottom of the page.
<!-- Bootstrap core JavaScript ================================================= --> <!-- Placed at the end of the document so the pages load faster --> <script src="https://code.jquery.com/jquery-3.1.1.slim.min.js" integrity="sha384-A7FZj7v+d/sdmMqp/nOQwliLvUsJfDHW+k9Omg/a/EheAdgtzNs3hpfag6Ed950n" crossorigin="anonymous"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/tether/1.4.0/js/tether.min.js" integrity="sha384-DztdAPBWPRXSA/3eYEEUWrWCy7G5KFbe8fFjk5JAIxUYHKkDx6Qin1DkWx51bBrb" crossorigin="anonymous"></script> <script src="https://maxcdn.bootstrapcdn.com/bootstrap/4.0.0-alpha.6/js/bootstrap.min.js" integrity="sha384-vBWWzlZJ8ea9aCX4pEW3rVHjgjt7zpkNpZk+02D9phzyeVkE+jo0ieGizqPLForn" crossorigin="anonymous"></script>
After creating all the views files, the views folder should look something like this:
That’s all there is to it. The sample Bootstrap template, Album Bootstrap, is now successfully integrated with Laravel.
Step 2: Test User Interface
Let’s create a view file that extends this layout file to see the Bootstrap layout integration with the Laravel Blade
template in action. Create a file demo.blade.php in the views folder and add the following content to the file:
@extends('layout.mainlayout') @section('content') <div class="album text-muted"> <div class="container"> <div class="row"> <h1>This is a demo text</h1> <p>Write any text to fill spaces</p> </div> </div> </div> @endsection
Step 3: Create the Routes
Now that the view is created, the next step is creating a route to access the view. For this, go to routes/web.php and paste the following routes.
Route::get('/demo', function () { return view('demo'); });
Now run the application using the staging URL. You will see the Album Bootstrap template integrated with your application.
Installing Bootstrap Template in Laravel
In this section, I’ll show how to integrate the CoolAdmin Dashboard into a Laravel app and how easy it is to add Bootstrap themes. I’ll also show how to integrate an admin theme step-by-step, a key feature for managing website components from the dashboard.
After installing the template, I’ll create two pages, i.e., my-home and my-users. This process shows the practical application of the integrated theme in creating functional web pages.
Downloading Dashboard With Laravel
First, choose and download a dashboard you’d like to use with your Laravel app. For example, the CoolAdmin Dashboard is user-friendly and easy to use. Once downloaded, extract the JS, CSS files, and icons and place them in your Laravel application.
Next, create a ‘theme’ folder within the ‘public’ folder of your Laravel application. Copy all the extracted data into this ‘theme’ directory.
Step 1: Config Route
First, you’ll configure the routes. Add two routes, my-home, and my-users, for the two pages. You can do this in the route file with the following code:
// routes/web.php Route::get('my-home', 'HomeController@myHome'); Route::get('my-users', 'HomeController@myUsers');
Step 2: Add Controller
Next, you’ll need to create a new HomeController and add two methods for the dashboard page and the user’s page. You can use the following code for the HomeController.
// app/Http/Controllers/HomeController.php <?php namespace App\Http\Controllers; use Illuminate\Http\Request; use App\Item; class HomeController extends Controller { /** * Create a new controller instance. * * @return void */ public function __construct() { } /** * Show the application dashboard. * * @return \Illuminate\Http\Response */ public function myHome() { return view('myHome'); } /** * Show the my users page. * * @return \Illuminate\Http\Response */ public function myUsers() { return view('myUsers'); } }
Step 3: Set up Theme Files
Next, you’ll set up theme blade files. For the CoolAdmin Dashboard theme, you’ll create three files. If you’re using a larger theme, you might need to create more files to manage it. In the ‘view’ folder, create a ‘theme’ folder that contains three files:
- default.blade.php
- header.blade.php
- sidebar.blade.php
You’ll then add code to these three files. For example, the path to the default file would be resources/views/theme/default.blade.php.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge"> <meta name="viewport" content="width=device-width, initial-scale=1"> <meta name="description" content=""> <meta name="author" content=""> <title>SB Admin 2 - Bootstrap Admin Theme</title> <!-- Bootstrap Core CSS --> <link href="{!! asset('theme/vendor/bootstrap/css/bootstrap.min.css') !!}" rel="stylesheet"> <!-- MetisMenu CSS --> <link href="{!! asset('theme/vendor/metisMenu/metisMenu.min.css') !!}" rel="stylesheet"> <!-- Custom CSS --> <link href="{!! asset('theme/dist/css/sb-admin-2.css') !!}" rel="stylesheet"> <!-- Morris Charts CSS --> <link href="{!! asset('theme/vendor/morrisjs/morris.css') !!}" rel="stylesheet"> <!-- Custom Fonts --> <link href="{!! asset('theme/vendor/font-awesome/css/font-awesome.min.css') !!}" rel="stylesheet" type="text/css"> </head> <body> <div id="wrapper"> <!-- Navigation --> <nav class="navbar navbar-default navbar-static-top" role="navigation" style="margin-bottom: 0"> @include('theme.header') @include('theme.sidebar') </nav> <div id="page-wrapper"> @yield('content') </div> <!-- /#page-wrapper --> </div> <!-- /#wrapper --> <!-- jQuery --> <script src="{!! asset('theme/vendor/jquery/jquery.min.js') !!}"></script> <!-- Bootstrap Core JavaScript --> <script src="{!! asset('theme/vendor/bootstrap/js/bootstrap.min.js') !!}"></script> <!-- Metis Menu Plugin JavaScript --> <script src="{!! asset('theme/vendor/metisMenu/metisMenu.min.js') !!}"></script> <!-- Morris Charts JavaScript --> <script src="{!! asset('theme/vendor/raphael/raphael.min.js') !!}"></script> <script src="{!! asset('theme/vendor/morrisjs/morris.min.js') !!}"></script> <script src="{!! asset('theme/data/morris-data.js') !!}"></script> <!-- Custom Theme JavaScript --> <script src="{!! asset('theme/dist/js/sb-admin-2.js') !!}"></script> </body> </html>
Step 4: Set up the Header
Insert the following code inside the directory file resources/views/theme/header.blade.php.
<nav class="navbar navbar-expand-lg navbar-transparent navbar-absolute fixed-top "> <div class="container-fluid"> <div class="navbar-wrapper"> <a class="navbar-brand" href="#pablo">Dashboard</a> </div> <button class="navbar-toggler" type="button" data-toggle="collapse" aria-controls="navigation-index" aria-expanded="false" aria-label="Toggle navigation"> <span class="sr-only">Toggle navigation</span> <span class="navbar-toggler-icon icon-bar"></span> <span class="navbar-toggler-icon icon-bar"></span> <span class="navbar-toggler-icon icon-bar"></span> </button> <div class="collapse navbar-collapse justify-content-end"> <form class="navbar-form"> <span class="bmd-form-group"> <div class="input-group no-border"> <input type="text" value="" class="form-control" placeholder="Search..."> <button type="submit" class="btn btn-white btn-round btn-just-icon"> <i class="material-icons">search</i> <div class="ripple-container"></div> </button> </div> </span> </form> <ul class="navbar-nav"> <li class="nav-item"> <a class="nav-link" href="#pablo"> <i class="material-icons">dashboard</i> <p class="d-lg-none d-md-block"> Stats </p> </a> </li> <li class="nav-item dropdown"> <a class="nav-link" href="http://example.com" id="navbarDropdownMenuLink" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false"> <i class="material-icons">notifications</i> <span class="notification">5</span> <p class="d-lg-none d-md-block"> Some Actions </p> </a> <div class="dropdown-menu dropdown-menu-right" aria-labelledby="navbarDropdownMenuLink"> <a class="dropdown-item" href="#">Mike John responded to your email</a> <a class="dropdown-item" href="#">You have 5 new tasks</a> <a class="dropdown-item" href="#">You're now friend with Andrew</a> <a class="dropdown-item" href="#">Another Notification</a> <a class="dropdown-item" href="#">Another One</a> </div> </li> <li class="nav-item dropdown"> <a class="nav-link" href="#pablo" id="navbarDropdownProfile" data-toggle="dropdown" aria-haspopup="true" aria-expanded="false"> <i class="material-icons">person</i> <p class="d-lg-none d-md-block"> Account </p> </a> <div class="dropdown-menu dropdown-menu-right" aria-labelledby="navbarDropdownProfile"> <a class="dropdown-item" href="#">Profile</a> <a class="dropdown-item" href="#">Settings</a> <div class="dropdown-divider"></div> <a class="dropdown-item" href="#">Log out</a> </div> </li> </ul> </div> </div> </nav>
Step 5: Set up the Sidebar
After successfully setting up the header, it’s time to set up the sidebar. Insert the following code.
<div class="sidebar" data-color="purple" data-background-color="white" data-image="../assets/img/sidebar-1.jpg"> <div class="logo"> <a href="http://www.creative-tim.com" class="simple-text logo-normal"> Creative Tim </a> </div> <div class="sidebar-wrapper ps-container ps-theme-default" data-ps-id="d8631889-919e-0705-2d4b-159fdbdfe8cd"> <ul class="nav"> <li class="nav-item active "> <a class="nav-link" href="./dashboard.html"> <i class="material-icons">dashboard</i> <p>Dashboard</p> </a> </li> <!-- More list items... --> </ul> </div> <div class="sidebar-background" style="background-image: url(../assets/img/sidebar-1.jpg) "></div> </div>
Step 6: Set up the Home
In this step, you’ll create two new blade files that use the integrated theme layout. You’ve added two routes, one for home
and another for users. Now, create two files to see how the integrated theme works.
The path to the home file, for example, would be resources/views/myHome.blade.php.
@extends('theme.default') @section('content') @endsection
Step 7: Employee Table
This table will be a part of the myUsers blade file and will display key information about each employee, such as their ID, name, salary, and country. Insert the following code into your myUsers.blade.php file.
<div class="col-lg-6 col-md-12"> <div class="card"> <div class="card-header card-header-warning"> <h4 class="card-title">Employees Stats</h4> <p class="card-category">New employees on 15th September, 2016</p> </div> <div class="card-body table-responsive"> <table class="table table-hover"> <thead class="text-warning"> <tr> <th>ID</th> <th>Name</th> <th>Salary</th> <th>Country</th> </tr> </thead> <tbody> <tr> <td>1</td> <td>Dakota Rice</td> <td>$36,738</td> <td>Niger</td> </tr> <tr> <td>2</td> <td>Minerva Hooper</td> <td>$23,789</td> <td>Curaçao</td> </tr> <tr> <td>3</td> <td>Sage Rodriguez</td> <td>$56,142</td> <td>Netherlands</td> </tr> <tr> <td>4</td> <td>Philip Chaney</td> <td>$38,735</td> <td>Korea, South</td> </tr> </tbody> </table> </div> </div> </div>
And there you have it!
By following these steps, you’ve successfully integrated the CoolAdmin Dashboard into your Laravel application and created a user-friendly interface with ‘home’ and ‘users’ pages.
Best Bootstrap Templates for Laravel
1. Vuexy – Laravel Admin Dashboard Template
Designed to streamline the process of building sophisticated and responsive web applications, Vuexy offers a wide array of pre-built components, widgets, and layout options, making it a go-to choice for creating modern and professional admin panels.
Vuexy has a modular and highly customizable architecture. It offers a clean and intuitive user interface that enhances the overall user experience, allowing developers to adapt the template to their specific project requirements effortlessly.
2. Argon Dashboard 2 Pro Laravel
Argon Dashboard 2 Pro Laravel is a full-stack app with Laravel that helps you build beautiful and functional web projects faster. It combines hundreds of Bootstrap 5 UI components with a Laravel backend with all the features you need for an admin panel.
You can choose from 54 example pages and 16 customized plugins to create your own web app. Argon Dashboard 2 Pro Laravel is easy to use and well-documented. It also supports SaaS applications where you can charge your users with a subscription plan.
3. Connect Plus Vue
Connect Plus Vue is a unique and beautifully designed admin dashboard template that combines power and simplicity. It has many UI elements and features, making it a versatile tool for any project. It stands out for its usability, typography, and design.
The template is backed by a dedicated support team, ensuring smooth and hassle-free usage. Whether you’re a seasoned developer or a beginner, Connect Plus Vue is a reliable choice for creating efficient and visually appealing admin dashboards.
Experience the Ease of Bootstrap Laravel Integration with Cloudways!
With support for all system requirements, from PHP 7.3 or higher, Composer, MySQL, Apache, Node.js, NPM, to Bootstrap, we’ve got you covered.
Summary
Integrating a Bootstrap template with Laravel is not only feasible but also highly beneficial. It allows developers to leverage the robustness of Laravel’s back-end capabilities with the responsiveness and aesthetic appeal of Bootstrap’s front-end components.
This guide simplifies the process of integrating Bootstrap with Laravel, making a complex task manageable. It enhances your efficiency and application aesthetics, leading to a smoother development experience. Happy integration!
1. How can I integrate a Bootstrap template into my Laravel project?
A. To integrate a Bootstrap template into a Laravel project, you need to first download the Bootstrap template. Then, cut the HTML into smaller Blade templates and include these in your main Blade file. You can use npm to install Bootstrap and Laravel’s frontend packages.
2. What are the benefits of using Bootstrap with Laravel?
A. Using Bootstrap with Laravel provides several benefits. Bootstrap offers user-friendly UI components that are responsive and compatible with different browsers. Laravel makes it easy to use these templates in your project’s views.
3. What are some best practices for integrating Bootstrap with Laravel?
A. Some best practices include creating a master template and extending it in your other templates. Keep different parts of the page in separate files for easy management. Install Bootstrap using npm and compile the assets to use in Laravel blade templates.
Shahzeb Ahmed
Shahzeb is a Digital Marketer with a Software Engineering background, works as a Community Manager — PHP Community at Cloudways. He is growth ambitious and aims to learn & share information about PHP & Laravel Development through practice and experimentation. He loves to travel and explore new ideas whenever he finds time. Get in touch with him at [email protected]