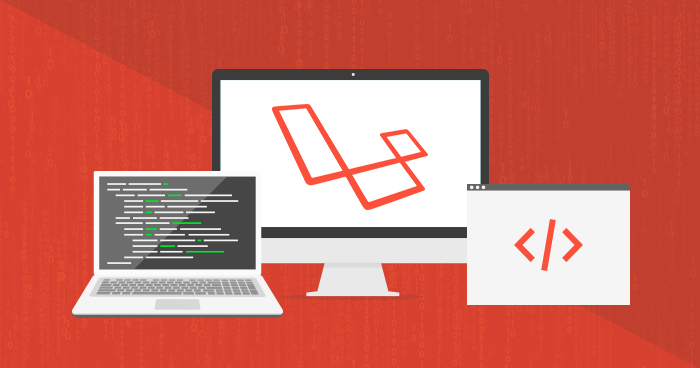
In this article, I will discuss Laravel API token authentication, a very important topic in web app and website security. I will demonstrate the basis of API token authentication and how easily you could implement the idea in your project.
The API authentication handle and validates the identity of the users attempting to make a connection by utilizing an authentication protocol. The protocol sends the credentials from the farther client asking the connection to the remote access server in either content or encrypted form.
What is API Token Authentication
The traditional process of interacting with a website is that you login from the login page. Next, you perform you desired actions and then log out. However, in the case of REST API, the process is quite different. The traditional procedure does not work in the case of RESTful APIs because the methods used on login page does not make any sense. You need to use api_token instead.
All you need to do is to append the api_token to the query string before making a request and the  request is authenticated.
Now what Laravel 5.5 offers is quite interesting! You can easily implement this idea using the Passport library.
By default, Laravel comes with a simple arrangement for API authentication of a token that is assigned to each user of the application. In config/auth. php setup file, an API guard is as of now characterized and uses a token driver.
To demonstrate the idea, I will make a simple application, in which users can register themselves, and by using their api tokens, will try to post some data into the database.
Stop Wasting Time on Servers
Cloudways handle server management for you so you can focus on creating great apps and keeping your clients happy.
Prerequisites
For the purpose of this tutorial, I assume that you have a Laravel application installed on a web server. My setup is:
- Laravel 5.5
- PHP 7.0
- MySQL
Since I don’t want o get sidetracked by server management during this tutorial, I decided to host the test app on a Cloudways managed server. In addition to a great hosting stack, they also take care of server management issues and provide pre installed Git and Composer. I suggest you sign up for a free account and then follow this GIF for setting up the server and the Laravel application.
Laravel Passport
Install Laravel Passport package in your application using run following command
composer require laravel/passport=~4.0
After install successfully Passport package in our Laravel application i need to set their Service Provider. so, open your config/app.php file and add following Line in the ‘providers’
Laravel\Passport\PassportServiceProvider::class,
Configure the Database
Now setup the MySQL database and configure it in Laravel.
In the project root, you will find the .env and config/database.php files. Add the database credentials (username, DB name, and password) to setup the database and allow the Laravel app access it.
Migrations
After set providers array now run the migration command
Php artisan migrate
Install Laravel Passport
After Migrations of tables is successfully completed now run the install passport command
:public_html$ php artisan passport:install
Configuration of  Passport
After install of Passport , next move to next Three step to configure it .
First open app/User.php file and update with following code.
namespace App; use Laravel\Passport\HasApiTokens; use Illuminate\Notifications\Notifiable; use Illuminate\Foundation\Auth\User as Authenticatable; class User extends Authenticatable {    use HasApiTokens, Notifiable;    /**     * The attributes that are mass assignable.     *     * @var array     */    protected $fillable = [        'name', 'email', 'password',    ];    /**     * The attributes that should be hidden for arrays.     *     * @var array     */    protected $hidden = [        'password', 'remember_token',    ]; }
Second go to the app/Providers/AuthServiceProvider.php  and update following code
namespace App\Providers; use Laravel\Passport\Passport; use Illuminate\Support\Facades\Gate; use Illuminate\Foundation\Support\Providers\AuthServiceProvider as ServiceProvider; class AuthServiceProvider extends ServiceProvider {    /**     * The policy mappings for the application.     *     * @var array     */    protected $policies = [        'App\Model' => 'App\Policies\ModelPolicy',    ];    /**     * Register any authentication / authorization services.     *     * @return void     */    public function boot()    {        $this->registerPolicies();        Passport::routes();    } }
Stop Wasting Time on Servers
Cloudways handle server management for you so you can focus on creating great apps and keeping your clients happy.
Third update the config/auth.php with following code
return [ Â 'guards' => [ Â Â Â Â Â Â Â 'web' => [ Â Â Â Â Â Â Â Â Â Â Â 'driver' => 'session', Â Â Â Â Â Â Â Â Â Â Â 'provider' => 'users', Â Â Â Â Â Â Â ], Â Â Â Â Â Â Â 'api' => [ Â Â Â Â Â Â Â Â Â Â Â 'driver' => 'passport', Â Â Â Â Â Â Â Â Â Â Â 'provider' => 'users', Â Â Â Â Â Â Â ], Â Â Â ], ]
Laravel AuthÂ
$ php artisan make:auth
Set API Route
Go in routes folder and open api.php
Route::middleware('auth:api')->get('/user', function (Request $request) { Â Â Â return $request->user(); }); Route::post('login', 'API\PassportController@login'); Route::post('register', 'API\PassportController@register'); Route::group(['middleware' => 'auth:api'], function(){ Route::post('get-details', 'API\PassportController@getDetails'); });
Create the Controller
create one PassportController.php controller in app/Http/Controllers/API/ root.
namespace App\Http\Controllers\API; use Illuminate\Http\Request; use App\Http\Controllers\Controller; use App\User; use Illuminate\Support\Facades\Auth; use Validator; class PassportController extends Controller {    public $successStatus = 200;    /**     * login api     *     * @return \Illuminate\Http\Response     */    public function login(){        if(Auth::attempt(['email' => request('email'), 'password' => request('password')])){            $user = Auth::user();            $success['token'] =  $user->createToken('MyApp')->accessToken;            return response()->json(['success' => $success], $this->successStatus);        }        else{            return response()->json(['error'=>'Unauthorised'], 401);        }    }    /**     * Register api     *     * @return \Illuminate\Http\Response     */    public function register(Request $request)    {        $validator = Validator::make($request->all(), [            'name' => 'required',            'email' => 'required|email',            'password' => 'required',            'c_password' => 'required|same:password',        ]);        if ($validator->fails()) {            return response()->json(['error'=>$validator->errors()], 401);                   }        $input = $request->all();        $input['password'] = bcrypt($input['password']);        $user = User::create($input);        $success['token'] =  $user->createToken('MyApp')->accessToken;        $success['name'] =  $user->name;        return response()->json(['success'=>$success], $this->successStatus);    }    /**     * details api     *     * @return \Illuminate\Http\Response     */    public function getDetails()    {        $user = Auth::user();        return response()->json(['success' => $user], $this->successStatus);    } }
Testing the App
Finally, run the php artisan server command and check application.The first step is to register a user. To do this, visit the URL of the application.
Test all API in any API testing tool, Â like Postman testing API and others tools.
Conclusion
In this tutorial, I demonstrated how you could use api_token for setting up secure communication within your app. If you would like to clarify a point discussed above or would like to extend the conversation, please leave a comment below.
Shahzeb Ahmed
Shahzeb is a Digital Marketer with a Software Engineering background, works as a Community Manager — PHP Community at Cloudways. He is growth ambitious and aims to learn & share information about PHP & Laravel Development through practice and experimentation. He loves to travel and explore new ideas whenever he finds time. Get in touch with him at [email protected]